RelativeLayout
是一种视图组,用于以相对位置显示子视图。每个视图的位置可以相对于同级元素(例如,另一个视图的左侧或下方)或相对于父 RelativeLayout
区域(例如,对齐到底部、左侧或中心)来指定。
注意:为了获得更好的性能和工具支持,您应该改为 使用 ConstraintLayout 构建您的布局。
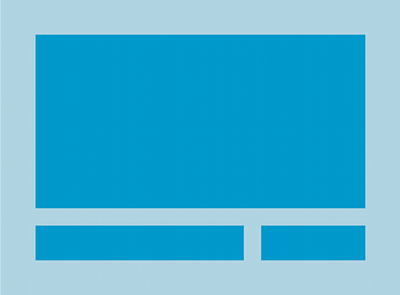
RelativeLayout
对于设计用户界面来说是一个非常强大的实用程序,因为它可以消除嵌套视图组并使您的布局层次结构保持扁平,从而提高性能。如果您发现自己使用了多个嵌套的 LinearLayout
组,则可以使用单个 RelativeLayout
来替换它们。
定位视图
RelativeLayout
允许子视图指定其相对于父视图或彼此(由 ID 指定)的位置。因此,您可以通过右侧边框对齐两个元素,或使一个元素位于另一个元素下方,位于屏幕中央、左侧居中,等等。默认情况下,所有子视图都绘制在布局的左上角,因此您必须使用 RelativeLayout.LayoutParams
提供的各种布局属性来定义每个视图的位置。
在 RelativeLayout
中,视图可用的许多布局属性中的一些包括
android:layout_alignParentTop
- 如果
"true"
,则使此视图的顶部边缘与父视图的顶部边缘匹配。 android:layout_centerVertical
- 如果
"true"
,则在此视图的父视图中垂直居中。 android:layout_below
- 将此视图的顶部边缘定位在使用资源 ID 指定的视图下方。
android:layout_toRightOf
- 将此视图的左边缘定位在使用资源 ID 指定的视图右侧。
这些只是一些示例。所有布局属性都在 RelativeLayout.LayoutParams
中有记录。
每个布局属性的值要么是一个布尔值,用于启用相对于父 RelativeLayout
的布局位置,要么是一个 ID,该 ID 引用布局中另一个视图,相对于该视图应定位该视图。
在您的 XML 布局中,可以按任何顺序声明对布局中其他视图的依赖项。例如,您可以声明“view1”应位于“view2”下方,即使“view2”是在层次结构中最后声明的视图。以下示例演示了这种情况。
示例
控制每个视图相对位置的每个属性都已加粗。
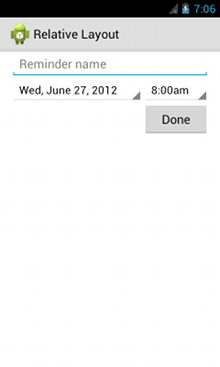
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="16dp" android:paddingRight="16dp" > <EditText android:id="@+id/name" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/reminder" /> <Spinner android:id="@+id/dates" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_below="@id/name" android:layout_alignParentLeft="true" android:layout_toLeftOf="@+id/times" /> <Spinner android:id="@id/times" android:layout_width="96dp" android:layout_height="wrap_content" android:layout_below="@id/name" android:layout_alignParentRight="true" /> <Button android:layout_width="96dp" android:layout_height="wrap_content" android:layout_below="@id/times" android:layout_alignParentRight="true" android:text="@string/done" /> </RelativeLayout>
有关 RelativeLayout
的每个子视图可用的所有布局属性的详细信息,请参阅 RelativeLayout.LayoutParams
。