有时您的布局需要很少使用的复杂视图。无论是项目详情、进度指示器还是撤消消息,您都可以在仅需要时加载视图,从而减少内存使用并加快渲染速度。
您可以通过为复杂且很少使用的视图定义 ViewStub
,来延迟加载应用将来需要的复杂视图资源。
定义 ViewStub
ViewStub
是一种轻量级视图,没有尺寸,不绘制任何内容,也不参与布局。因此,在视图层次结构中加载和保留它需要的资源很少。每个 ViewStub
都包含 android:layout
属性,用于指定要加载的布局。
假设您有一个布局,希望在用户旅程的后期加载
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:src="@drawable/logo" android:layout_width="match_parent" android:layout_height="match_parent"/> </FrameLayout>
您可以使用以下 ViewStub
推迟加载。要使其显示或加载任何内容,您必须使其显示引用的布局
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/root" android:layout_width="match_parent" android:layout_height="match_parent"> <ViewStub android:id="@+id/stub_import" android:inflatedId="@+id/panel_import" android:layout="@layout/heavy_layout_we_want_to_postpone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="bottom" /> </FrameLayout>
加载 ViewStub 布局
上一节中的代码片段会产生类似于图 1 的效果
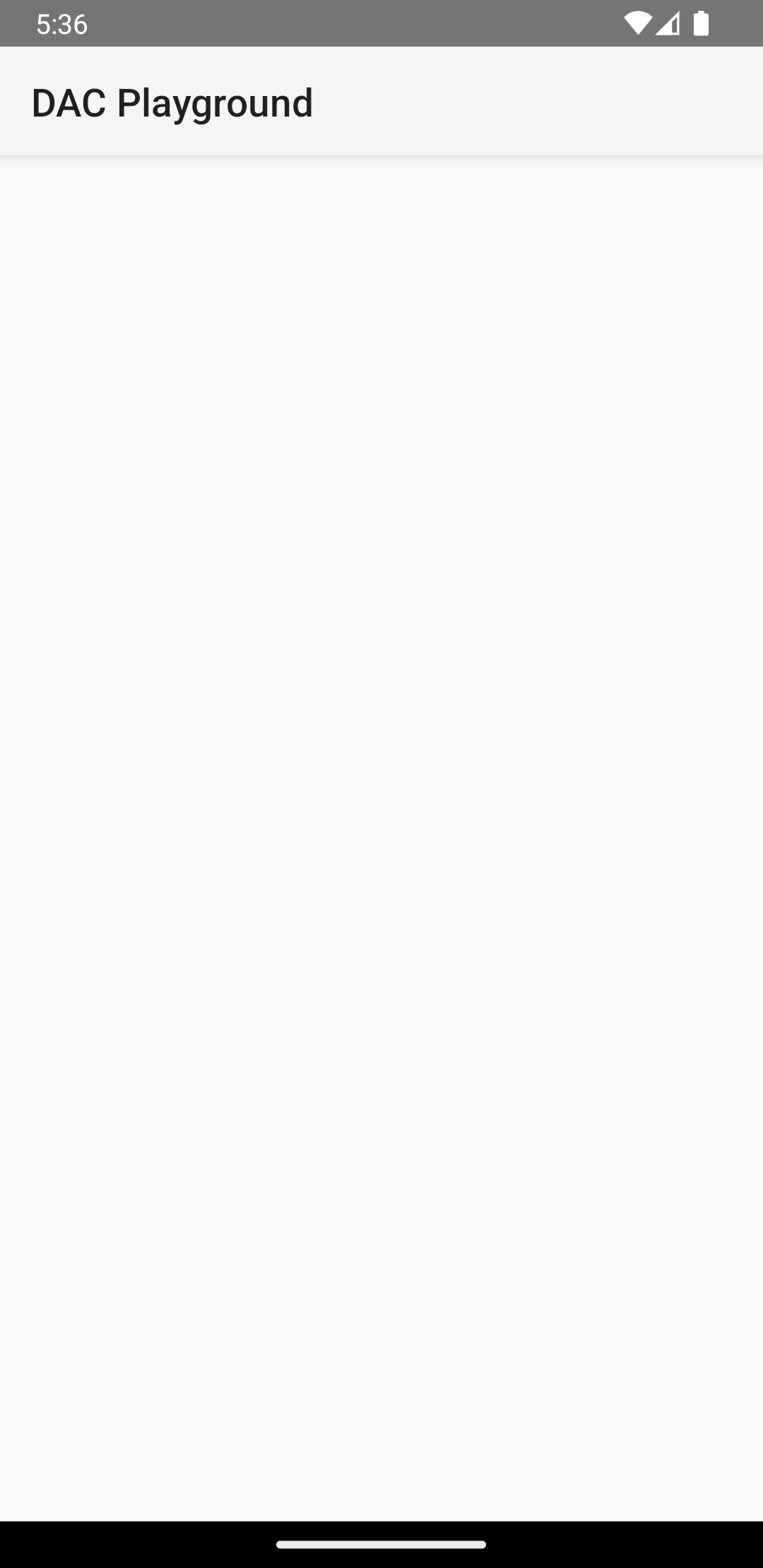
ViewStub
隐藏了复杂的布局。当您想加载 ViewStub
指定的布局时,可以通过调用 setVisibility(View.VISIBLE)
使其可见,或调用 inflate()
。
以下代码片段模拟了延迟加载。屏幕在 Activity
和 onCreate()
中像往常一样加载,然后显示 heavy_layout_we_want_to_postpone
布局
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_old_xml) Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000) }
Java
@Override void onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_old_xml); Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000); }
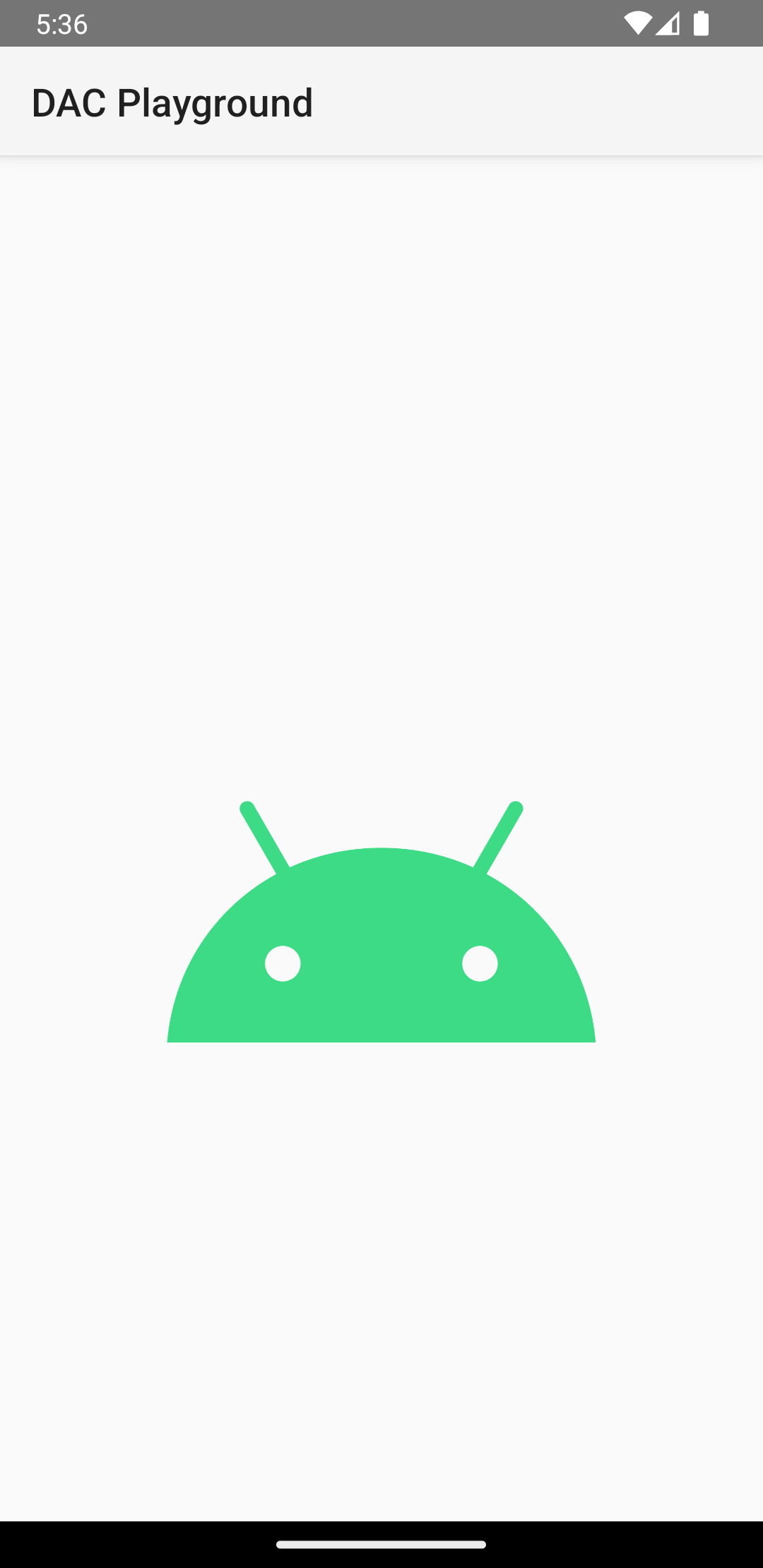
一旦可见或加载,ViewStub
元素就不再是视图层次结构的一部分。它被加载的布局替换,并且该布局根视图的 ID 由 ViewStub
的 android:inflatedId
属性指定。ViewStub
指定的 ID android:id
仅在 ViewStub
布局可见或加载之前有效。
有关此主题的更多信息,请参阅博客文章 使用 stub 进行优化。