在 OpenGL ES 环境中,投影和相机视图可以让你以一种更接近肉眼观察物理对象的方式显示绘制的对象。这种物理观察的模拟是通过对绘制对象的坐标进行数学变换来实现的。
- 投影 - 这种变换根据显示对象的
GLSurfaceView
的宽度和高度调整绘制对象的坐标。如果没有这种计算,OpenGL ES 绘制的对象就会因为视图窗口的不等比例而发生扭曲。投影变换通常只需在渲染器的onSurfaceChanged()
方法中确定或更改 OpenGL 视图的比例时进行计算。有关 OpenGL ES 投影和坐标映射的更多信息,请参阅为绘制的对象映射坐标。 - 相机视图 - 这种变换根据虚拟相机的位置调整绘制对象的坐标。请务必注意,OpenGL ES 并未定义实际的相机对象,而是提供了通过变换绘制对象的显示来模拟相机功能的实用方法。相机视图变换可能只在确定
GLSurfaceView
时计算一次,也可能根据用户操作或应用的功能进行动态更改。
本课程介绍如何创建投影和相机视图,并将其应用于在 GLSurfaceView
中绘制的形状。
定义投影
投影变换的数据在 GLSurfaceView.Renderer
类的 onSurfaceChanged()
方法中计算。以下示例代码获取 GLSurfaceView
的高和宽,并使用 Matrix.frustumM()
方法来填充投影变换 Matrix
:
Kotlin
// vPMatrix is an abbreviation for "Model View Projection Matrix" private val vPMatrix = FloatArray(16) private val projectionMatrix = FloatArray(16) private val viewMatrix = FloatArray(16) override fun onSurfaceChanged(unused: GL10, width: Int, height: Int) { GLES20.glViewport(0, 0, width, height) val ratio: Float = width.toFloat() / height.toFloat() // this projection matrix is applied to object coordinates // in the onDrawFrame() method Matrix.frustumM(projectionMatrix, 0, -ratio, ratio, -1f, 1f, 3f, 7f) }
Java
// vPMatrix is an abbreviation for "Model View Projection Matrix" private final float[] vPMatrix = new float[16]; private final float[] projectionMatrix = new float[16]; private final float[] viewMatrix = new float[16]; @Override public void onSurfaceChanged(GL10 unused, int width, int height) { GLES20.glViewport(0, 0, width, height); float ratio = (float) width / height; // this projection matrix is applied to object coordinates // in the onDrawFrame() method Matrix.frustumM(projectionMatrix, 0, -ratio, ratio, -1, 1, 3, 7); }
此代码填充一个投影矩阵 mProjectionMatrix
,然后你可以在 onDrawFrame()
方法中将其与相机视图变换结合起来,如下一节所示。
注意:只对绘制对象应用投影变换通常会导致屏幕显示非常空旷。通常情况下,你还需要应用相机视图变换,屏幕上才会显示任何内容。
定义相机视图
通过在渲染器的绘制过程中添加相机视图变换,完成对绘制对象的变换。在下面的示例代码中,使用 Matrix.setLookAtM()
方法计算相机视图变换,然后将其与先前计算的投影矩阵相结合。接着将组合后的变换矩阵传递给绘制的形状。
Kotlin
override fun onDrawFrame(unused: GL10) { ... // Set the camera position (View matrix) Matrix.setLookAtM(viewMatrix, 0, 0f, 0f, 3f, 0f, 0f, 0f, 0f, 1.0f, 0.0f) // Calculate the projection and view transformation Matrix.multiplyMM(vPMatrix, 0, projectionMatrix, 0, viewMatrix, 0) // Draw shape triangle.draw(vPMatrix)
Java
@Override public void onDrawFrame(GL10 unused) { ... // Set the camera position (View matrix) Matrix.setLookAtM(viewMatrix, 0, 0, 0, 3, 0f, 0f, 0f, 0f, 1.0f, 0.0f); // Calculate the projection and view transformation Matrix.multiplyMM(vPMatrix, 0, projectionMatrix, 0, viewMatrix, 0); // Draw shape triangle.draw(vPMatrix); }
应用投影和相机变换
为了使用前面几节中显示的组合投影和相机视图变换矩阵,首先要将一个矩阵变量添加到之前在 Triangle
类中定义的顶点着色器中:
Kotlin
class Triangle { private val vertexShaderCode = // This matrix member variable provides a hook to manipulate // the coordinates of the objects that use this vertex shader "uniform mat4 uMVPMatrix;" + "attribute vec4 vPosition;" + "void main() {" + // the matrix must be included as a modifier of gl_Position // Note that the uMVPMatrix factor *must be first* in order // for the matrix multiplication product to be correct. " gl_Position = uMVPMatrix * vPosition;" + "}" // Use to access and set the view transformation private var vPMatrixHandle: Int = 0 ... }
Java
public class Triangle { private final String vertexShaderCode = // This matrix member variable provides a hook to manipulate // the coordinates of the objects that use this vertex shader "uniform mat4 uMVPMatrix;" + "attribute vec4 vPosition;" + "void main() {" + // the matrix must be included as a modifier of gl_Position // Note that the uMVPMatrix factor *must be first* in order // for the matrix multiplication product to be correct. " gl_Position = uMVPMatrix * vPosition;" + "}"; // Use to access and set the view transformation private int vPMatrixHandle; ... }
接下来,修改图形对象的 draw()
方法,以接受组合后的变换矩阵并将其应用于形状:
Kotlin
fun draw(mvpMatrix: FloatArray) { // pass in the calculated transformation matrix ... // get handle to shape's transformation matrix vPMatrixHandle = GLES20.glGetUniformLocation(mProgram, "uMVPMatrix") // Pass the projection and view transformation to the shader GLES20.glUniformMatrix4fv(vPMatrixHandle, 1, false, mvpMatrix, 0) // Draw the triangle GLES20.glDrawArrays(GLES20.GL_TRIANGLES, 0, vertexCount) // Disable vertex array GLES20.glDisableVertexAttribArray(positionHandle) }
Java
public void draw(float[] mvpMatrix) { // pass in the calculated transformation matrix ... // get handle to shape's transformation matrix vPMatrixHandle = GLES20.glGetUniformLocation(mProgram, "uMVPMatrix"); // Pass the projection and view transformation to the shader GLES20.glUniformMatrix4fv(vPMatrixHandle, 1, false, mvpMatrix, 0); // Draw the triangle GLES20.glDrawArrays(GLES20.GL_TRIANGLES, 0, vertexCount); // Disable vertex array GLES20.glDisableVertexAttribArray(positionHandle); }
正确计算并应用投影和相机视图变换后,图形对象将以正确的比例绘制,看起来应该像这样:
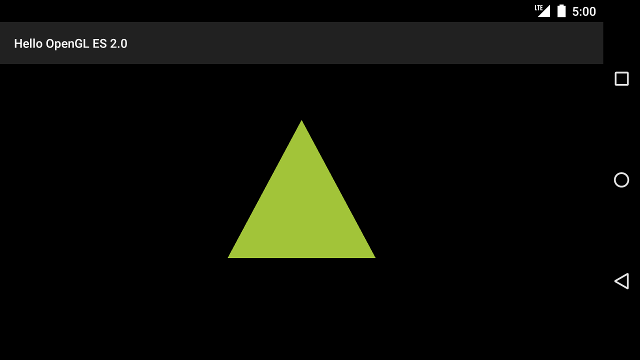
图 1. 应用了投影和相机视图绘制的三角形。
现在你已拥有一个按正确比例显示形状的应用,接下来可以为形状添加动作了。