列表使用户能够轻松地在Wear OS设备上从一组选项中选择一个项目。
Wearable UI库包含 WearableRecyclerView
类,它是用于创建针对可穿戴设备优化的列表的RecyclerView
实现。您可以通过创建一个新的WearableRecyclerView
容器在您的可穿戴应用中使用此界面。
对于简单的项目的较长列表(例如应用程序启动器或联系人列表),请使用WearableRecyclerView
。每个项目可能包含一个短字符串和一个关联的图标。或者,每个项目可能仅包含一个字符串或一个图标。
注意:避免使用复杂的布局。用户只需要看一眼项目就能理解它是什么,尤其是在可穿戴设备的屏幕尺寸有限的情况下。
通过扩展现有的RecyclerView
类,WearableRecyclerView
API默认显示一个垂直可滚动的项目直线列表。您还可以使用WearableRecyclerView
API选择启用曲线布局和圆形滚动手势,以在您的可穿戴应用中使用。
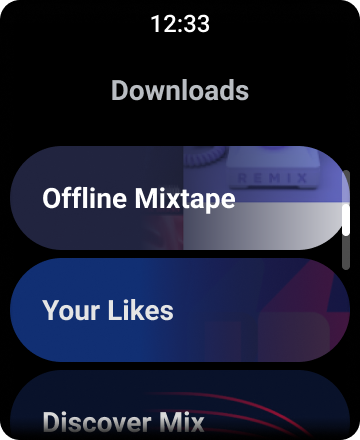
图 1.Wear OS上的默认列表视图。
本指南将向您展示如何使用WearableRecyclerView
类在Wear OS应用中创建列表,如何选择启用可滚动项目的曲线布局,以及如何在滚动时自定义子项的外观。
使用XML将WearableRecyclerView添加到活动
以下布局将WearableRecyclerView
添加到活动
<androidx.wear.widget.WearableRecyclerView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/recycler_launcher_view" android:layout_width="match_parent" android:layout_height="match_parent" android:scrollbars="vertical" />
以下示例演示了应用于 Activity 的WearableRecyclerView
Kotlin
class MainActivity : Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } ... }
Java
public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } ... }
创建弯曲布局
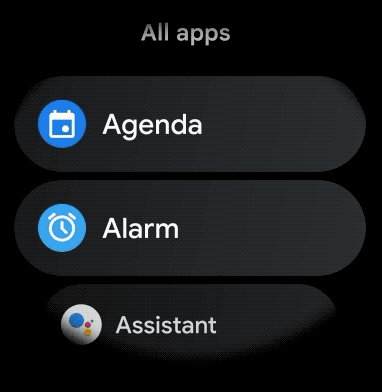
要在可穿戴应用中为可滚动项目创建弯曲布局,请执行以下操作
- 在相关的 XML 布局中,使用
WearableRecyclerView
作为主要容器。 - 将
setEdgeItemsCenteringEnabled(boolean)
方法设置为true
。这将在屏幕上垂直居中列表中的第一个和最后一个项目。 - 使用
WearableRecyclerView.setLayoutManager()
方法设置屏幕上项目的布局。
Kotlin
wearableRecyclerView.apply { // To align the edge children (first and last) with the center of the screen. isEdgeItemsCenteringEnabled = true ... layoutManager = WearableLinearLayoutManager(this@MainActivity) }
Java
// To align the edge children (first and last) with the center of the screen. wearableRecyclerView.setEdgeItemsCenteringEnabled(true); ... wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this));
如果您的应用有特定要求需要在滚动时自定义子项的外观(例如,在项目从中心滚动时缩放图标和文本),请扩展 WearableLinearLayoutManager.LayoutCallback
类并覆盖 onLayoutFinished
方法。
以下代码片段显示了一个自定义项目滚动以使其远离中心进一步缩放的示例,方法是扩展WearableLinearLayoutManager.LayoutCallback
类
Kotlin
/** How much icons should scale, at most. */ private const val MAX_ICON_PROGRESS = 0.65f class CustomScrollingLayoutCallback : WearableLinearLayoutManager.LayoutCallback() { private var progressToCenter: Float = 0f override fun onLayoutFinished(child: View, parent: RecyclerView) { child.apply { // Figure out % progress from top to bottom. val centerOffset = height.toFloat() / 2.0f / parent.height.toFloat() val yRelativeToCenterOffset = y / parent.height + centerOffset // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset) // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS) scaleX = 1 - progressToCenter scaleY = 1 - progressToCenter } } }
Java
public class CustomScrollingLayoutCallback extends WearableLinearLayoutManager.LayoutCallback { /** How much icons should scale, at most. */ private static final float MAX_ICON_PROGRESS = 0.65f; private float progressToCenter; @Override public void onLayoutFinished(View child, RecyclerView parent) { // Figure out % progress from top to bottom. float centerOffset = ((float) child.getHeight() / 2.0f) / (float) parent.getHeight(); float yRelativeToCenterOffset = (child.getY() / parent.getHeight()) + centerOffset; // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset); // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS); child.setScaleX(1 - progressToCenter); child.setScaleY(1 - progressToCenter); } }
Kotlin
wearableRecyclerView.layoutManager = WearableLinearLayoutManager(this, CustomScrollingLayoutCallback())
Java
CustomScrollingLayoutCallback customScrollingLayoutCallback = new CustomScrollingLayoutCallback(); wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this, customScrollingLayoutCallback));