内容提供方管理对中心数据仓库的访问。提供方是 Android 应用的一部分,它通常提供自己的 UI 来处理数据。但是,内容提供方主要由其他应用使用,这些应用使用提供方客户端对象访问提供方。提供方和提供方客户端一起提供一致、标准的数据接口,同时处理进程间通信和安全数据访问。
通常,您会在以下两种场景之一中使用内容提供方:实现代码以访问其他应用中现有的内容提供方,或在您的应用中创建新的内容提供方以与其他应用共享数据。
本页面介绍了使用现有内容提供方的基础知识。要了解如何在自己的应用中实现内容提供方,请参阅 创建内容提供方。
本主题介绍以下内容:
- 内容提供方的工作原理。
- 用于从内容提供方检索数据的 API。
- 用于在内容提供方中插入、更新或删除数据的 API。
- 有助于使用提供方的其他 API 功能。
概览
内容提供方向外部应用提供数据,形式为一个或多个表,这些表类似于关系数据库中的表。行代表提供方收集的某种类型数据的一个实例,行中的每一列代表为该实例收集的一条独立数据。
内容提供方协调应用中数据存储层的访问,以便许多不同的 API 和组件使用。如图 1 所示,这些内容包括:
- 与其他应用共享您的应用数据的访问权限
- 将数据发送到微件
- 通过搜索框架使用
SearchRecentSuggestionsProvider
返回应用的自定义搜索建议 - 使用
AbstractThreadedSyncAdapter
的实现将应用数据与服务器同步 - 使用
CursorLoader
在 UI 中加载数据
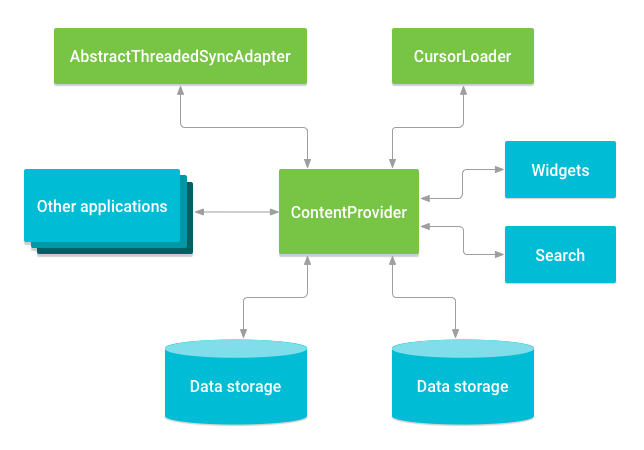
图 1. 内容提供方与其他组件之间的关系。
访问提供方
当您想访问内容提供方中的数据时,您可以使用应用 Context
中的 ContentResolver
对象,以客户端身份与提供方通信。ContentResolver
对象与提供方对象(实现 ContentProvider
接口的类实例)进行通信。
提供方对象接收来自客户端的数据请求,执行请求的操作,并返回结果。此对象具有方法,这些方法会调用提供方对象(ContentProvider
具体子类的实例)中同名的方法。ContentResolver
方法提供持久化存储的基本“CRUD”(创建、检索、更新和删除)函数。
从 UI 访问 ContentProvider
的常见模式是使用 CursorLoader
在后台运行异步查询。UI 中的 Activity
或 Fragment
调用 CursorLoader
来进行查询,CursorLoader
又使用 ContentResolver
获取 ContentProvider
。
这样,在查询运行时,UI 可以继续对用户可用。此模式涉及许多不同对象以及底层存储机制之间的交互,如图 2 所示。
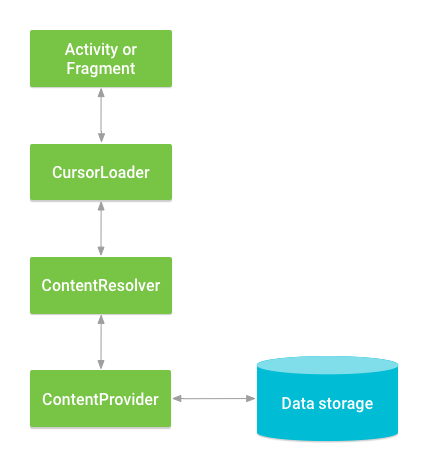
图 2. ContentProvider
、其他类和存储之间的交互。
注意:要访问提供方,您的应用通常必须在其清单文件中请求特定的权限。此开发模式在内容提供方权限部分中有更详细的描述。
Android 平台内置的提供方之一是用户字典提供方,它存储用户想保留的非标准词语。表 1 说明了此提供方的表中的数据可能是什么样子:
表 1:用户字典示例表。
word | app id | frequency | locale | _ID |
---|---|---|---|---|
mapreduce |
user1 | 100 | en_US | 1 |
precompiler |
user14 | 200 | fr_FR | 2 |
applet |
user2 | 225 | fr_CA | 3 |
const |
user1 | 255 | pt_BR | 4 |
int |
user5 | 100 | en_UK | 5 |
在表 1 中,每一行代表标准字典中未找到的词语的一个实例。每一列代表该词语的一条数据,例如首次遇到它的区域设置。列标题是提供方中存储的列名。因此,例如,要引用行的区域设置,您就引用其 locale
列。对于此提供方,_ID
列充当提供方自动维护的 primary key(主键)列。
要从用户字典提供方获取词语及其区域设置的列表,您可以调用 ContentResolver.query()
。query()
方法会调用用户字典提供方定义的 ContentProvider.query()
方法。以下代码行展示了 ContentResolver.query()
调用:
Kotlin
// Queries the UserDictionary and returns results cursor = contentResolver.query( UserDictionary.Words.CONTENT_URI, // The content URI of the words table projection, // The columns to return for each row selectionClause, // Selection criteria selectionArgs.toTypedArray(), // Selection criteria sortOrder // The sort order for the returned rows )
Java
// Queries the UserDictionary and returns results cursor = getContentResolver().query( UserDictionary.Words.CONTENT_URI, // The content URI of the words table projection, // The columns to return for each row selectionClause, // Selection criteria selectionArgs, // Selection criteria sortOrder); // The sort order for the returned rows
表 2 显示了 query(Uri,projection,selection,selectionArgs,sortOrder)
的参数如何与 SQL SELECT 语句匹配
表 2: query()
与 SQL 查询的对比。
query() 参数 |
SELECT 关键字/参数 | 说明 |
---|---|---|
Uri |
FROM table_name |
Uri 映射到提供方中名为 table_name 的表。 |
projection |
col,col,col,... |
projection 是一个列数组,包含在检索到的每一行中。 |
selection |
WHERE col = value |
selection 指定选择行的条件。 |
selectionArgs |
没有完全等效项。选择参数替换选择子句中的 ? 占位符。 |
|
sortOrder |
ORDER BY col,col,... |
sortOrder 指定返回的 Cursor 中行的排列顺序。 |
内容 URI
内容 URI 是用于标识提供方中数据的 URI。内容 URI 包括整个提供方的符号名称(其 authority)以及指向表的名称(path)。当您调用客户端方法访问提供方中的表时,该表的内容 URI 是参数之一。
在前面的代码行中,常量 CONTENT_URI
包含用户字典提供方的 Words
表的内容 URI。ContentResolver
对象会解析出 URI 的 authority,并通过将 authority 与已知提供方的系统表进行比较来 解析 提供方。ContentResolver
随后可以将查询参数分派给正确的提供方。
ContentProvider
使用内容 URI 的路径部分来选择要访问的表。提供方通常为其公开的每个表都有一个路径。
在前面的代码行中,Words
表的完整 URI 是
content://user_dictionary/words
content://
字符串是 scheme,始终存在,并将其标识为内容 URI。user_dictionary
字符串是提供方的 authority。words
字符串是表的路径。
许多提供方允许您通过在 URI 末尾附加 ID 值来访问表中的单行。例如,要从用户字典提供方检索 _ID
为 4
的行,您可以使用此内容 URI:
Kotlin
val singleUri: Uri = ContentUris.withAppendedId(UserDictionary.Words.CONTENT_URI, 4)
Java
Uri singleUri = ContentUris.withAppendedId(UserDictionary.Words.CONTENT_URI,4);
当您检索一组行并随后想更新或删除其中一行时,通常会使用 ID 值。
注意: Uri
和 Uri.Builder
类包含用于从字符串构造格式正确的 URI 对象的便捷方法。ContentUris
类包含用于将 ID 值附加到 URI 的便捷方法。前面的代码片段使用 withAppendedId()
将 ID 附加到用户字典提供方内容 URI。
从提供方检索数据
本部分介绍如何从提供方检索数据,并以用户字典提供方为例。
为了清晰起见,本节中的代码片段在 UI 线程上调用 ContentResolver.query()
。然而,在实际代码中,请在单独的线程上异步执行查询。您可以使用 CursorLoader
类,加载器指南中对此有更详细的介绍。此外,这些代码行只是片段。它们并未展示完整的应用。
要从提供方检索数据,请按照以下基本步骤操作:
- 请求提供方的读取访问权限。
- 定义向提供方发送查询的代码。
请求读取访问权限
要从提供方检索数据,您的应用需要提供方的读取访问权限。您无法在运行时请求此权限。相反,您必须在清单文件中使用 <uses-permission>
元素和提供方定义的准确权限名称来指定您需要此权限。
当您在清单中指定此元素时,您是在为您的应用请求此权限。当用户安装您的应用时,他们会隐式授予此请求。
要查找您正在使用的提供方的读取访问权限的准确名称以及提供方使用的其他访问权限的名称,请查阅提供方的文档。
权限在访问提供方中的作用在内容提供方权限部分中有更详细的描述。
用户字典提供方在其清单文件中定义了权限 android.permission.READ_USER_DICTIONARY
,因此想要从该提供方读取的应用必须请求此权限。
构造查询
从提供方检索数据的下一步是构造查询。以下代码片段定义了一些用于访问用户字典提供方的变量
Kotlin
// A "projection" defines the columns that are returned for each row private val mProjection: Array<String> = arrayOf( UserDictionary.Words._ID, // Contract class constant for the _ID column name UserDictionary.Words.WORD, // Contract class constant for the word column name UserDictionary.Words.LOCALE // Contract class constant for the locale column name ) // Defines a string to contain the selection clause private var selectionClause: String? = null // Declares an array to contain selection arguments private lateinit var selectionArgs: Array<String>
Java
// A "projection" defines the columns that are returned for each row String[] mProjection = { UserDictionary.Words._ID, // Contract class constant for the _ID column name UserDictionary.Words.WORD, // Contract class constant for the word column name UserDictionary.Words.LOCALE // Contract class constant for the locale column name }; // Defines a string to contain the selection clause String selectionClause = null; // Initializes an array to contain selection arguments String[] selectionArgs = {""};
以下代码片段展示了如何使用 ContentResolver.query()
,并以用户字典提供方为例。提供方客户端查询类似于 SQL 查询,它包含要返回的列集、选择条件集和排序顺序。
查询返回的列集称为 projection,变量为 mProjection
。
指定要检索的行的表达式被分为选择子句和选择参数。选择子句是逻辑表达式、布尔表达式、列名和值的组合。变量为 mSelectionClause
。如果您指定可替换参数 ?
而不是值,则查询方法将从选择参数数组(即变量 mSelectionArgs
)中检索值。
在下一个代码片段中,如果用户未输入词语,则选择子句设置为 null
,查询将返回提供方中的所有词语。如果用户输入词语,则选择子句设置为 UserDictionary.Words.WORD + " = ?"
,并且选择参数数组的第一个元素设置为用户输入的词语。
Kotlin
/* * This declares a String array to contain the selection arguments. */ private lateinit var selectionArgs: Array<String> // Gets a word from the UI searchString = searchWord.text.toString() // Insert code here to check for invalid or malicious input // If the word is the empty string, gets everything selectionArgs = searchString?.takeIf { it.isNotEmpty() }?.let { selectionClause = "${UserDictionary.Words.WORD} = ?" arrayOf(it) } ?: run { selectionClause = null emptyArray<String>() } // Does a query against the table and returns a Cursor object mCursor = contentResolver.query( UserDictionary.Words.CONTENT_URI, // The content URI of the words table projection, // The columns to return for each row selectionClause, // Either null or the word the user entered selectionArgs, // Either empty or the string the user entered sortOrder // The sort order for the returned rows ) // Some providers return null if an error occurs, others throw an exception when (mCursor?.count) { null -> { /* * Insert code here to handle the error. Be sure not to use the cursor! * You might want to call android.util.Log.e() to log this error. */ } 0 -> { /* * Insert code here to notify the user that the search is unsuccessful. This isn't * necessarily an error. You might want to offer the user the option to insert a new * row, or re-type the search term. */ } else -> { // Insert code here to do something with the results } }
Java
/* * This defines a one-element String array to contain the selection argument. */ String[] selectionArgs = {""}; // Gets a word from the UI searchString = searchWord.getText().toString(); // Remember to insert code here to check for invalid or malicious input // If the word is the empty string, gets everything if (TextUtils.isEmpty(searchString)) { // Setting the selection clause to null returns all words selectionClause = null; selectionArgs[0] = ""; } else { // Constructs a selection clause that matches the word that the user entered selectionClause = UserDictionary.Words.WORD + " = ?"; // Moves the user's input string to the selection arguments selectionArgs[0] = searchString; } // Does a query against the table and returns a Cursor object mCursor = getContentResolver().query( UserDictionary.Words.CONTENT_URI, // The content URI of the words table projection, // The columns to return for each row selectionClause, // Either null or the word the user entered selectionArgs, // Either empty or the string the user entered sortOrder); // The sort order for the returned rows // Some providers return null if an error occurs, others throw an exception if (null == mCursor) { /* * Insert code here to handle the error. Be sure not to use the cursor! You can * call android.util.Log.e() to log this error. * */ // If the Cursor is empty, the provider found no matches } else if (mCursor.getCount() < 1) { /* * Insert code here to notify the user that the search is unsuccessful. This isn't necessarily * an error. You can offer the user the option to insert a new row, or re-type the * search term. */ } else { // Insert code here to do something with the results }
此查询类似于以下 SQL 语句
SELECT _ID, word, locale FROM words WHERE word = <userinput> ORDER BY word ASC;
在此 SQL 语句中,使用了实际列名而不是契约类常量。
防范恶意输入
如果内容提供方管理的数据位于 SQL 数据库中,将外部不可信数据包含到原始 SQL 语句中可能会导致 SQL 注入。
考虑以下选择子句
Kotlin
// Constructs a selection clause by concatenating the user's input to the column name var selectionClause = "var = $mUserInput"
Java
// Constructs a selection clause by concatenating the user's input to the column name String selectionClause = "var = " + userInput;
如果您这样做,就允许用户将恶意 SQL 潜在地连接到您的 SQL 语句上。例如,用户可以为 mUserInput
输入 "nothing; DROP TABLE *;",这将导致选择子句变为 var = nothing; DROP TABLE *;
。
由于选择子句被视为 SQL 语句,这可能会导致提供方擦除底层 SQLite 数据库中的所有表,除非提供方已配置为捕获 SQL 注入尝试。
为了避免这个问题,请使用以 ?
作为可替换参数的选择子句以及单独的选择参数数组。这样,用户输入直接绑定到查询,而不是被解释为 SQL 语句的一部分。由于它不被视为 SQL,用户输入无法注入恶意 SQL。您应使用此选择子句来包含用户输入,而不是使用连接:
Kotlin
// Constructs a selection clause with a replaceable parameter var selectionClause = "var = ?"
Java
// Constructs a selection clause with a replaceable parameter String selectionClause = "var = ?";
按如下方式设置选择参数数组
Kotlin
// Defines a mutable list to contain the selection arguments var selectionArgs: MutableList<String> = mutableListOf()
Java
// Defines an array to contain the selection arguments String[] selectionArgs = {""};
按如下方式在选择参数数组中放置值
Kotlin
// Adds the user's input to the selection argument selectionArgs += userInput
Java
// Sets the selection argument to the user's input selectionArgs[0] = userInput;
使用以 ?
作为可替换参数的选择子句和选择参数数组是指定选择的首选方式,即使提供方不是基于 SQL 数据库。
显示查询结果
ContentResolver.query()
客户端方法始终返回一个 Cursor
,其中包含查询投影指定的、匹配查询选择条件的行的列。Cursor
对象提供对其包含的行和列的随机读取访问。
使用 Cursor
方法,您可以遍历结果中的行,确定每列的数据类型,从列中获取数据,以及检查结果的其他属性。
某些 Cursor
实现会在提供方数据更改时自动更新对象,并在 Cursor
更改时触发观察者对象中的方法,或者两者兼而有之。
注意:提供方可以根据进行查询的对象的性质来限制对列的访问。例如,联系人提供方限制某些列仅供同步适配器访问,因此它不会将这些列返回给 activity 或 service。
如果没有行匹配选择条件,提供方将返回一个 Cursor
对象,其 Cursor.getCount()
为 0,即空游标。
如果发生内部错误,查询结果取决于具体的提供方。它可能返回 null
,或者抛出 Exception
。
由于 Cursor
是行的列表,显示 Cursor
内容的好方法是使用 SimpleCursorAdapter
将其链接到 ListView
。
以下代码片段延续了前面的代码片段。它创建一个包含查询检索到的 Cursor
的 SimpleCursorAdapter
对象,并将此对象设置为 ListView
的适配器。
Kotlin
// Defines a list of columns to retrieve from the Cursor and load into an output row val wordListColumns : Array<String> = arrayOf( UserDictionary.Words.WORD, // Contract class constant containing the word column name UserDictionary.Words.LOCALE // Contract class constant containing the locale column name ) // Defines a list of View IDs that receive the Cursor columns for each row val wordListItems = intArrayOf(R.id.dictWord, R.id.locale) // Creates a new SimpleCursorAdapter cursorAdapter = SimpleCursorAdapter( applicationContext, // The application's Context object R.layout.wordlistrow, // A layout in XML for one row in the ListView mCursor, // The result from the query wordListColumns, // A string array of column names in the cursor wordListItems, // An integer array of view IDs in the row layout 0 // Flags (usually none are needed) ) // Sets the adapter for the ListView wordList.setAdapter(cursorAdapter)
Java
// Defines a list of columns to retrieve from the Cursor and load into an output row String[] wordListColumns = { UserDictionary.Words.WORD, // Contract class constant containing the word column name UserDictionary.Words.LOCALE // Contract class constant containing the locale column name }; // Defines a list of View IDs that receive the Cursor columns for each row int[] wordListItems = { R.id.dictWord, R.id.locale}; // Creates a new SimpleCursorAdapter cursorAdapter = new SimpleCursorAdapter( getApplicationContext(), // The application's Context object R.layout.wordlistrow, // A layout in XML for one row in the ListView mCursor, // The result from the query wordListColumns, // A string array of column names in the cursor wordListItems, // An integer array of view IDs in the row layout 0); // Flags (usually none are needed) // Sets the adapter for the ListView wordList.setAdapter(cursorAdapter);
注意:要使用 Cursor
支持 ListView
,游标必须包含一个名为 _ID
的列。因此,前面显示的查询检索了 Words
表的 _ID
列,尽管 ListView
不会显示它。此限制也解释了为什么大多数提供方对其每个表都有一个 _ID
列。
从查询结果中获取数据
除了显示查询结果外,您还可以将它们用于其他任务。例如,您可以从用户字典提供方检索拼写,然后到其他提供方中查找它们。为此,您需要遍历 Cursor
中的行,如以下示例所示:
Kotlin
/* * Only executes if the cursor is valid. The User Dictionary Provider returns null if * an internal error occurs. Other providers might throw an Exception instead of returning null. */ mCursor?.apply { // Determine the column index of the column named "word" val index: Int = getColumnIndex(UserDictionary.Words.WORD) /* * Moves to the next row in the cursor. Before the first movement in the cursor, the * "row pointer" is -1, and if you try to retrieve data at that position you get an * exception. */ while (moveToNext()) { // Gets the value from the column newWord = getString(index) // Insert code here to process the retrieved word ... // End of while loop } }
Java
// Determine the column index of the column named "word" int index = mCursor.getColumnIndex(UserDictionary.Words.WORD); /* * Only executes if the cursor is valid. The User Dictionary Provider returns null if * an internal error occurs. Other providers might throw an Exception instead of returning null. */ if (mCursor != null) { /* * Moves to the next row in the cursor. Before the first movement in the cursor, the * "row pointer" is -1, and if you try to retrieve data at that position you get an * exception. */ while (mCursor.moveToNext()) { // Gets the value from the column newWord = mCursor.getString(index); // Insert code here to process the retrieved word ... // End of while loop } } else { // Insert code here to report an error if the cursor is null or the provider threw an exception }
Cursor
实现包含几个“get”方法,用于从对象中检索不同类型的数据。例如,前面的代码片段使用了 getString()
。它们还有一个 getType()
方法,该方法返回一个值,指示列的数据类型。
释放查询结果资源
不再需要 Cursor
对象时必须将其关闭,以便更快地释放与之相关的资源。这可以通过调用 close()
完成,或者在 Java 编程语言中使用 try-with-resources
语句,在 Kotlin 编程语言中使用 use()
函数完成。
内容提供方权限
提供方的应用可以指定其他应用必须拥有的权限才能访问提供方的数据。这些权限让用户知道应用尝试访问哪些数据。根据提供方的要求,其他应用会请求它们访问提供方所需的权限。最终用户在安装应用时会看到请求的权限。
如果提供方的应用未指定任何权限,则其他应用无权访问提供方的数据,除非提供方已导出。此外,提供方应用中的组件始终具有完整的读写访问权限,无论指定的权限是什么。
用户字典提供方需要 android.permission.READ_USER_DICTIONARY
权限才能从中检索数据。该提供方具有单独的 android.permission.WRITE_USER_DICTIONARY
权限,用于插入、更新或删除数据。
要获取访问提供方所需的权限,应用在其清单文件中使用 <uses-permission>
元素请求这些权限。当 Android Package Manager 安装应用时,用户必须批准应用请求的所有权限。如果用户批准,Package Manager 会继续安装。如果用户不批准,Package Manager 会停止安装。
以下示例 <uses-permission>
元素请求对用户字典提供方的读取访问权限
<uses-permission android:name="android.permission.READ_USER_DICTIONARY">
权限对提供方访问的影响在安全提示中有更详细的解释。
插入、更新和删除数据
与从提供方检索数据的方式相同,您也可以使用提供方客户端与提供方的 ContentProvider
之间的交互来修改数据。您调用 ContentResolver
的方法,并传递将传递给 ContentProvider
的相应方法的参数。提供方和提供方客户端会自动处理安全性和进程间通信。
插入数据
要将数据插入到提供方,您可以调用 ContentResolver.insert()
方法。此方法将新行插入到提供方并返回该行的内容 URI。以下代码片段展示了如何将新词语插入用户字典提供方:
Kotlin
// Defines a new Uri object that receives the result of the insertion lateinit var newUri: Uri ... // Defines an object to contain the new values to insert val newValues = ContentValues().apply { /* * Sets the values of each column and inserts the word. The arguments to the "put" * method are "column name" and "value". */ put(UserDictionary.Words.APP_ID, "example.user") put(UserDictionary.Words.LOCALE, "en_US") put(UserDictionary.Words.WORD, "insert") put(UserDictionary.Words.FREQUENCY, "100") } newUri = contentResolver.insert( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI newValues // The values to insert )
Java
// Defines a new Uri object that receives the result of the insertion Uri newUri; ... // Defines an object to contain the new values to insert ContentValues newValues = new ContentValues(); /* * Sets the values of each column and inserts the word. The arguments to the "put" * method are "column name" and "value". */ newValues.put(UserDictionary.Words.APP_ID, "example.user"); newValues.put(UserDictionary.Words.LOCALE, "en_US"); newValues.put(UserDictionary.Words.WORD, "insert"); newValues.put(UserDictionary.Words.FREQUENCY, "100"); newUri = getContentResolver().insert( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI newValues // The values to insert );
新行的数据进入单个 ContentValues
对象,其形式类似于单行游标。此对象中的列无需具有相同的数据类型,如果您根本不想指定值,可以使用 ContentValues.putNull()
将列设置为 null
。
前面的代码片段没有添加 _ID
列,因为此列是自动维护的。提供方为添加的每一行分配唯一的 _ID
值。提供方通常将此值用作表的主键。
newUri
中返回的内容 URI 标识了新添加的行,格式如下:
content://user_dictionary/words/<id_value>
<id_value>
是新行 _ID
的内容。大多数提供方可以自动检测这种形式的内容 URI,然后对特定行执行请求的操作。
要从返回的 Uri
中获取 _ID
的值,请调用 ContentUris.parseId()
。
更新数据
要更新行,您使用带有更新值的 ContentValues
对象,就像插入时一样,并使用选择条件,就像查询时一样。您使用的客户端方法是 ContentResolver.update()
。您只需为要更新的列向 ContentValues
对象添加值。如果要清除列的内容,请将值设置为 null
。
以下代码片段将所有区域设置语言为 "en"
的行的区域设置更改为 null
。返回值为更新的行数。
Kotlin
// Defines an object to contain the updated values val updateValues = ContentValues().apply { /* * Sets the updated value and updates the selected words. */ putNull(UserDictionary.Words.LOCALE) } // Defines selection criteria for the rows you want to update val selectionClause: String = UserDictionary.Words.LOCALE + "LIKE ?" val selectionArgs: Array<String> = arrayOf("en_%") // Defines a variable to contain the number of updated rows var rowsUpdated: Int = 0 ... rowsUpdated = contentResolver.update( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI updateValues, // The columns to update selectionClause, // The column to select on selectionArgs // The value to compare to )
Java
// Defines an object to contain the updated values ContentValues updateValues = new ContentValues(); // Defines selection criteria for the rows you want to update String selectionClause = UserDictionary.Words.LOCALE + " LIKE ?"; String[] selectionArgs = {"en_%"}; // Defines a variable to contain the number of updated rows int rowsUpdated = 0; ... /* * Sets the updated value and updates the selected words. */ updateValues.putNull(UserDictionary.Words.LOCALE); rowsUpdated = getContentResolver().update( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI updateValues, // The columns to update selectionClause, // The column to select on selectionArgs // The value to compare to );
在调用 ContentResolver.update()
时清理用户输入。要了解更多信息,请阅读防范恶意输入部分。
删除数据
删除行类似于检索行数据。您指定要删除的行的选择条件,客户端方法返回删除的行数。以下代码片段删除应用 ID 匹配 "user"
的行。该方法返回删除的行数。
Kotlin
// Defines selection criteria for the rows you want to delete val selectionClause = "${UserDictionary.Words.APP_ID} LIKE ?" val selectionArgs: Array<String> = arrayOf("user") // Defines a variable to contain the number of rows deleted var rowsDeleted: Int = 0 ... // Deletes the words that match the selection criteria rowsDeleted = contentResolver.delete( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI selectionClause, // The column to select on selectionArgs // The value to compare to )
Java
// Defines selection criteria for the rows you want to delete String selectionClause = UserDictionary.Words.APP_ID + " LIKE ?"; String[] selectionArgs = {"user"}; // Defines a variable to contain the number of rows deleted int rowsDeleted = 0; ... // Deletes the words that match the selection criteria rowsDeleted = getContentResolver().delete( UserDictionary.Words.CONTENT_URI, // The UserDictionary content URI selectionClause, // The column to select on selectionArgs // The value to compare to );
在调用 ContentResolver.delete()
时清理用户输入。要了解更多信息,请阅读防范恶意输入部分。
提供方数据类型
内容提供方可以提供许多不同的数据类型。用户字典提供方仅提供文本,但提供方也可以提供以下格式:
- 整型 (integer)
- 长整型 (long)
- 浮点型 (floating point)
- 长浮点型 (double)
提供方常使用的另一种数据类型是二进制大对象 (BLOB),实现为 64 KB 字节数组。您可以通过查看 Cursor
类的“get”方法来查看可用的数据类型。
提供方中每列的数据类型通常列在其文档中。用户字典提供方的数据类型列在其契约类 UserDictionary.Words
的参考文档中。契约类在契约类部分中进行了描述。您也可以通过调用 Cursor.getType()
来确定数据类型。
提供方还维护其定义的每个内容 URI 的 MIME 数据类型信息。您可以使用 MIME 类型信息来了解您的应用是否可以处理提供方提供的数据,或根据 MIME 类型选择处理方式。当您使用包含复杂数据结构或文件的提供方时,通常需要 MIME 类型。
例如,联系人提供方中的 ContactsContract.Data
表使用 MIME 类型标记每行中存储的联系人数据类型。要获取与内容 URI 相对应的 MIME 类型,请调用 ContentResolver.getType()
。
从MIME 类型参考部分描述了标准和自定义 MIME 类型的语法。
提供方访问的其他形式
在应用开发中,有三种重要的替代提供方访问形式:
-
批量访问:您可以使用
ContentProviderOperation
类中的方法创建一批访问调用,然后使用ContentResolver.applyBatch()
应用它们。 - 异步查询:在单独的线程中执行查询。您可以使用
CursorLoader
对象。加载器指南中的示例演示了如何执行此操作。 - 使用 Intent 访问数据:虽然您无法直接向提供方发送 intent,但您可以向提供方的应用发送 intent,该应用通常最适合修改提供方的数据。
以下部分将介绍批量访问和使用 intent 进行修改。
批量访问
批量访问提供方对于插入大量行、在同一方法调用中向多个表插入行以及通常跨进程边界执行一组操作(称为 atomic operation)非常有用。
要以批量模式访问提供方,请创建一个 ContentProviderOperation
对象数组,然后使用 ContentResolver.applyBatch()
将其分派给内容提供方。您将内容提供方的 authority 传递给此方法,而不是特定的内容 URI。
这使得数组中的每个 ContentProviderOperation
对象都能操作不同的表。调用 ContentResolver.applyBatch()
会返回一个结果数组。
ContactsContract.RawContacts
契约类的描述包含一个展示批量插入的代码片段。
使用 Intent 访问数据
Intent 可以提供对内容提供方的间接访问。即使您的应用没有访问权限,您也可以通过从具有权限的应用获取结果 intent,或者通过激活具有权限的应用并允许用户在其中进行操作来让用户访问提供方中的数据。
通过临时权限获取访问权限
您可以访问内容提供方中的数据,即使您没有适当的访问权限,方法是将 intent 发送到具有权限的应用,并接收包含 URI 权限的结果 intent。这些是针对特定内容 URI 的权限,有效期直到接收它们的 Activity 结束。具有永久权限的应用通过在结果 intent 中设置标志来授予临时权限:
注意:这些标志不提供对内容 URI 中包含 authority 的提供方的通用读写访问。访问仅限于 URI 本身。
将内容 URI 发送给其他应用时,请至少包含这些标志之一。这些标志为接收 intent 并以 Android 11(API 级别 30)或更高版本为目标的任何应用提供了以下功能:
- 根据 intent 中包含的标志,读取或写入内容 URI 代表的数据。
- 获得包含与 URI authority 匹配的内容提供方应用的包可见性。发送 intent 的应用和包含内容提供方的应用可能是两个不同的应用。
提供方使用 <provider>
元素的 android:grantUriPermissions
属性以及 <provider>
元素的子元素 <grant-uri-permission>
在其清单中为内容 URI 定义 URI 权限。URI 权限机制在Android 上的权限指南中有更详细的解释。
例如,即使您没有 READ_CONTACTS
权限,您也可以检索联系人提供方中联系人的数据。您可能希望在向联系人发送生日电子贺卡的应用中执行此操作。与其请求 READ_CONTACTS
权限(这会使您访问用户的所有联系人及其所有信息),不如让用户控制您的应用使用哪些联系人。为此,请使用以下流程:
- 在您的应用中,使用方法
startActivityForResult()
发送包含操作ACTION_PICK
和“contacts”MIME 类型CONTENT_ITEM_TYPE
的 intent。 - 由于此 intent 匹配联系人应用的“选择”activity 的 intent 过滤器,该 activity 会显示在前台。
- 在选择 activity 中,用户选择要更新的联系人。发生此情况时,选择 activity 调用
setResult(resultcode, intent)
来设置 intent 返回给您的应用。intent 包含用户选择的联系人的内容 URI 以及“extras”标志FLAG_GRANT_READ_URI_PERMISSION
。这些标志授予您的应用读取内容 URI 指向的联系人数据的 URI 权限。然后选择 activity 调用finish()
将控制权返回给您的应用。 - 您的 activity 返回前台,系统调用您的 activity 的
onActivityResult()
方法。此方法接收联系人应用中选择 activity 创建的结果 intent。 - 通过结果 intent 中的内容 URI,您可以从联系人提供方读取联系人的数据,即使您未在清单中请求对提供方的永久读取访问权限。然后您可以获取联系人的生日信息或电子邮件地址,然后发送电子贺卡。
使用其他应用
让用户修改您没有访问权限的数据的另一种方法是激活具有权限的应用,然后让用户在那里进行操作。
例如,日历应用接受一个 ACTION_INSERT
intent,允许您激活应用的插入 UI。您可以在此 intent 中传递“extras”数据,应用会使用这些数据预填充 UI。由于重复事件的语法很复杂,将事件插入日历提供方的首选方法是使用 ACTION_INSERT
激活日历应用,然后让用户在那里插入事件。
使用辅助应用显示数据
如果您的应用确实具有访问权限,您仍然可以使用 intent 在其他应用中显示数据。例如,日历应用接受一个 ACTION_VIEW
intent,用于显示特定日期或事件。这让您可以显示日历信息,而无需创建自己的 UI。要了解有关此功能的更多信息,请参阅日历提供方概览。
您发送 intent 的应用不必是与提供方关联的应用。例如,您可以从联系人提供方检索联系人,然后将包含联系人图片内容 URI 的 ACTION_VIEW
intent 发送给图片查看器。
契约类
契约类定义了常量,这些常量有助于应用使用内容 URI、列名、intent 操作以及内容提供方的其他功能。契约类不会随提供方自动包含。提供方的开发者必须定义它们,然后将其提供给其他开发者。Android 平台包含的许多提供方在 android.provider
包中都有相应的契约类。
例如,用户字典提供方有一个契约类 UserDictionary
,其中包含内容 URI 和列名常量。Words
表的内容 URI 在常量 UserDictionary.Words.CONTENT_URI
中定义。UserDictionary.Words
类还包含列名常量,本指南中的示例代码片段中使用了这些常量。例如,查询投影可以定义如下:
Kotlin
val projection : Array<String> = arrayOf( UserDictionary.Words._ID, UserDictionary.Words.WORD, UserDictionary.Words.LOCALE )
Java
String[] projection = { UserDictionary.Words._ID, UserDictionary.Words.WORD, UserDictionary.Words.LOCALE };
另一个契约类是联系人提供方的 ContactsContract
。此类的参考文档包含示例代码片段。其子类之一 ContactsContract.Intents.Insert
是一个契约类,其中包含 intent 和 intent 数据的常量。
MIME 类型参考
内容提供方可以返回标准的 MIME 媒体类型、自定义 MIME 类型字符串或两者兼而有之。
MIME 类型的格式如下:
type/subtype
例如,众所周知的 MIME 类型 text/html
具有 text
类型和 html
子类型。如果提供方为此 URI 返回此类型,则表示使用该 URI 的查询返回包含 HTML 标记的文本。
自定义 MIME 类型字符串,也称为 vendor-specific(供应商特定)MIME 类型,具有更复杂的 type 和 subtype 值。对于多行,类型值始终如下:
vnd.android.cursor.dir
对于单行,类型值始终如下:
vnd.android.cursor.item
The subtype is provider-specific. The Android built-in providers usually have a simple subtype. For example, when the Contacts application creates a row for a telephone number, it sets the following MIME type in the row
vnd.android.cursor.item/phone_v2
子类型值为 phone_v2
。
其他提供方开发者可以基于提供方的 authority 和表名创建自己的子类型模式。例如,考虑一个包含火车时刻表的提供方。提供方的 authority 是 com.example.trains
,它包含表 Line1、Line2 和 Line3。响应表 Line1 的以下内容 URI 时:
content://com.example.trains/Line1
提供方返回以下 MIME 类型:
vnd.android.cursor.dir/vnd.example.line1
响应表 Line2 中第 5 行的以下内容 URI 时:
content://com.example.trains/Line2/5
提供方返回以下 MIME 类型:
vnd.android.cursor.item/vnd.example.line2
大多数内容提供方都为其使用的 MIME 类型定义了契约类常量。例如,联系人提供方契约类 ContactsContract.RawContacts
定义了常量 CONTENT_ITEM_TYPE
,用于表示单个原始联系人行的 MIME 类型。
单行的内容 URI 在内容 URI部分中进行了描述。