Android 提供各种工具和 API,可帮助您创建针对不同屏幕和窗口尺寸的测试。
DeviceConfigurationOverride
使用 DeviceConfigurationOverride
可组合项,您可以覆盖配置属性以测试 Compose 布局中的多个屏幕和窗口尺寸。 ForcedSize
覆盖适合可用空间中的任何布局,这使您可以对任何屏幕尺寸运行任何 UI 测试。例如,您可以使用小型手机外形规格来运行所有 UI 测试,包括大型手机、折叠屏手机和平板电脑的 UI 测试。
DeviceConfigurationOverride(
DeviceConfigurationOverride.ForcedSize(DpSize(1280.dp, 800.dp))
) {
MyScreen() // Will be rendered in the space for 1280dp by 800dp without clipping.
}
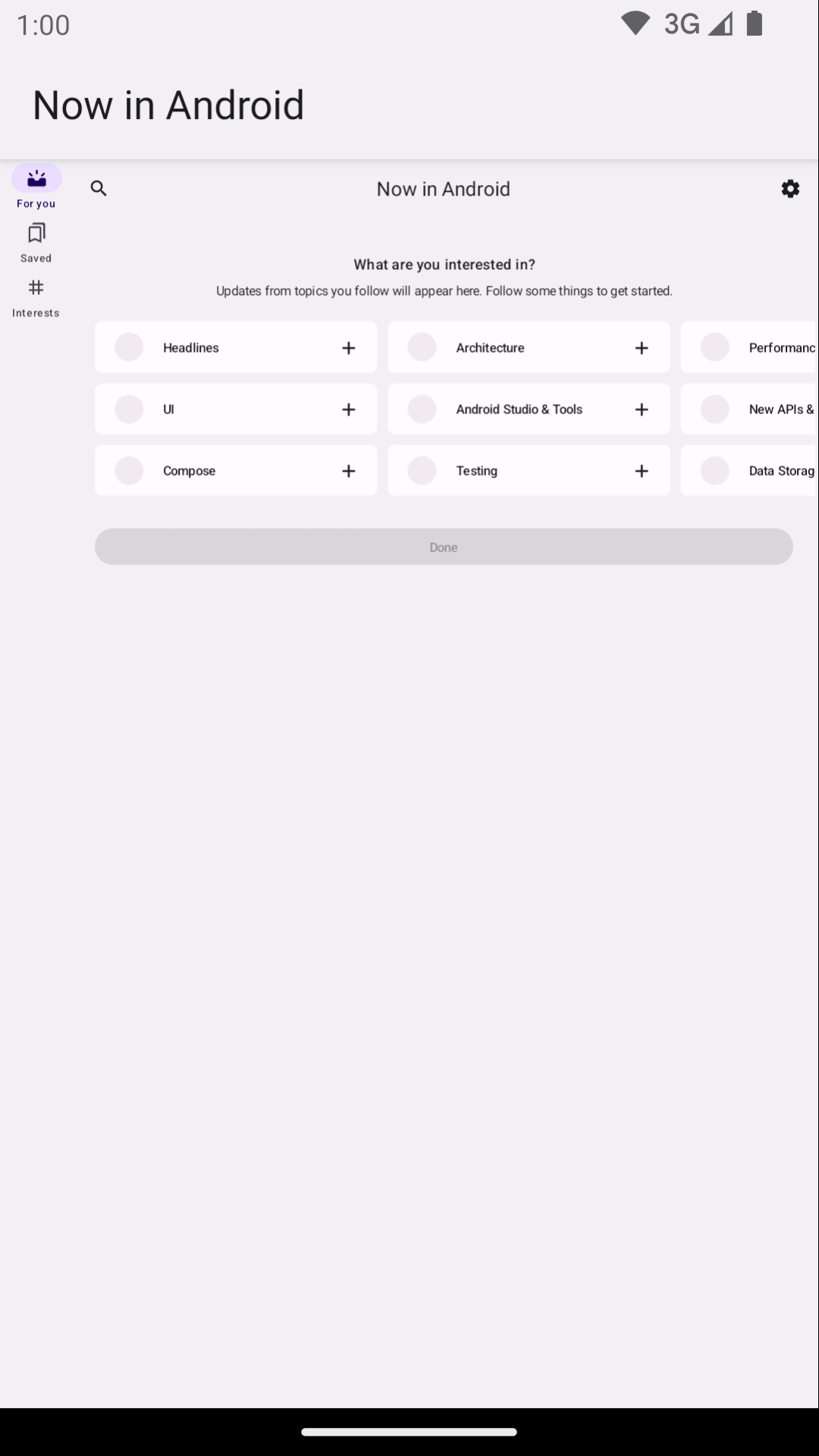
此外,您还可以使用此可组合项来设置字体比例、主题以及您可能想要在不同窗口尺寸上测试的其他属性。
Robolectric
使用 Robolectric 在 JVM 上 本地 运行 Compose 或基于视图的 UI 测试,无需设备或模拟器。您可以 配置 Robolectric 以使用特定屏幕尺寸以及其他有用的属性。
在来自 *Android 最新动态* 的以下 示例 中,Robolectric 配置为模拟 1000x1000 dp 的屏幕尺寸,分辨率为 480 dpi。
@RunWith(RobolectricTestRunner::class)
// Configure Robolectric to use a very large screen size that can fit all of the test sizes.
// This allows enough room to render the content under test without clipping or scaling.
@Config(qualifiers = "w1000dp-h1000dp-480dpi")
class NiaAppScreenSizesScreenshotTests { ... }
您还可以像 *Android 最新动态* 示例中的此代码片段一样,从测试主体设置限定符
val (width, height, dpi) = ...
// Set qualifiers from specs.
RuntimeEnvironment.setQualifiers("w${width}dp-h${height}dp-${dpi}dpi")
请注意,RuntimeEnvironment.setQualifiers()
会使用新配置更新系统和应用程序资源,但不会对活动活动或其他组件触发任何操作。
您可以在 Robolectric 的 设备配置 文档中阅读更多信息。
Gradle 托管设备
Gradle 托管设备 (GMD) Android Gradle 插件允许您定义模拟器和运行 工具化 测试的真实设备的规格。为具有不同屏幕尺寸的设备创建规格,以实现必须在特定屏幕尺寸上运行某些测试的测试策略。通过将 GMD 与 持续集成 (CI) 结合使用,您可以确保在需要时运行适当的测试,预配和启动模拟器并简化您的 CI 设置。
android {
testOptions {
managedDevices {
devices {
// Run with ./gradlew nexusOneApi30DebugAndroidTest.
nexusOneApi30(com.android.build.api.dsl.ManagedVirtualDevice) {
device = "Nexus One"
apiLevel = 30
// Use the AOSP ATD image for better emulator performance
systemImageSource = "aosp-atd"
}
// Run with ./gradlew foldApi34DebugAndroidTest.
foldApi34(com.android.build.api.dsl.ManagedVirtualDevice) {
device = "Pixel Fold"
apiLevel = 34
systemImageSource = "aosp-atd"
}
}
}
}
}
您可以在 testing-samples 项目中找到多个 GMD 示例。
Firebase 测试实验室
使用 Firebase 测试实验室 (FTL) 或类似的设备农场服务,在您可能无法访问的特定真实设备(例如各种尺寸的折叠屏手机和平板电脑)上运行测试。Firebase 测试实验室是一项 付费服务,并提供免费层级。FTL 还支持在模拟器上运行测试。这些服务可以提高工具化测试的可靠性和速度,因为它们可以提前预配设备和模拟器。
有关将 FTL 与 GMD 结合使用的信息,请参阅 使用 Gradle 托管设备扩展测试规模。
使用测试运行程序进行测试筛选
最佳测试策略不应重复验证同一内容,因此大多数 UI 测试无需在多台设备上运行。通常,您可以通过在手机外形规格上运行所有或大多数 UI 测试,并且仅在具有不同屏幕尺寸的设备上运行子集来筛选 UI 测试。
您可以为某些测试添加注释,使其仅在某些设备上运行,然后使用运行测试的命令将参数传递给 AndroidJUnitRunner。
例如,您可以创建不同的注释
annotation class TestExpandedWidth
annotation class TestCompactWidth
并在不同的测试中使用它们
class MyTestClass {
@Test
@TestExpandedWidth
fun myExample_worksOnTablet() {
...
}
@Test
@TestCompactWidth
fun myExample_worksOnPortraitPhone() {
...
}
}
然后,在运行测试时,您可以使用 android.testInstrumentationRunnerArguments.annotation
属性来筛选特定的测试。例如,如果您使用 Gradle 托管设备
$ ./gradlew pixelTabletApi30DebugAndroidTest -Pandroid.testInstrumentationRunnerArguments.annotation='com.sample.TestExpandedWidth'
如果您不使用 GMD 并管理 CI 上的模拟器,请首先确保正确的模拟器或设备已准备好并已连接,然后将参数传递给运行工具化测试的 Gradle 命令之一
$ ./gradlew connectedAndroidTest -Pandroid.testInstrumentationRunnerArguments.annotation='com.sample.TestExpandedWidth'
请注意,Espresso 设备(请参见下一部分)也可以使用设备属性筛选测试。
Espresso 设备
使用 Espresso 设备 在测试中对模拟器执行操作,使用任何类型的工具化测试,包括 Espresso、Compose 或 UI Automator 测试。这些操作可能包括设置屏幕尺寸或切换折叠状态或姿势。例如,您可以控制可折叠模拟器并将其设置为桌面模式。Espresso 设备还包含 JUnit 规则和注释,以要求某些功能
@RunWith(AndroidJUnit4::class)
class OnDeviceTest {
@get:Rule(order=1) val activityScenarioRule = activityScenarioRule<MainActivity>()
@get:Rule(order=2) val screenOrientationRule: ScreenOrientationRule =
ScreenOrientationRule(ScreenOrientation.PORTRAIT)
@Test
fun tabletopMode_playerIsDisplayed() {
// Set the device to tabletop mode.
onDevice().setTabletopMode()
onView(withId(R.id.player)).check(matches(isDisplayed()))
}
}
请注意,Espresso 设备 仍处于 alpha 阶段,并具有以下要求
- Android Gradle 插件 8.3 或更高版本
- Android 模拟器 33.1.10 或更高版本
- 运行 API 级别 24 或更高的 Android 虚拟设备
过滤测试
Espresso Device 可以读取已连接设备的属性,使您可以使用注解过滤测试。如果未满足带注解的要求,则会跳过测试。
RequiresDeviceMode 注解
RequiresDeviceMode
注解可以多次使用,以指示只有在设备支持所有DeviceMode
值时才运行的测试。
class OnDeviceTest {
...
@Test
@RequiresDeviceMode(TABLETOP)
@RequiresDeviceMode(BOOK)
fun tabletopMode_playerIdDisplayed() {
// Set the device to tabletop mode.
onDevice().setTabletopMode()
onView(withId(R.id.player)).check(matches(isDisplayed()))
}
}
RequiresDisplay 注解
RequiresDisplay
注解允许您使用尺寸类别指定设备屏幕的宽度和高度,这些尺寸类别根据官方的窗口尺寸类别定义尺寸范围。
class OnDeviceTest {
...
@Test
@RequiresDisplay(EXPANDED, COMPACT)
fun myScreen_expandedWidthCompactHeight() {
...
}
}
调整显示尺寸
使用setDisplaySize()
方法在运行时调整屏幕的尺寸。将此方法与DisplaySizeRule
类结合使用,该类确保在下一个测试之前撤消测试期间所做的任何更改。
@RunWith(AndroidJUnit4::class)
class ResizeDisplayTest {
@get:Rule(order = 1) val activityScenarioRule = activityScenarioRule<MainActivity>()
// Test rule for restoring device to its starting display size when a test case finishes.
@get:Rule(order = 2) val displaySizeRule: DisplaySizeRule = DisplaySizeRule()
@Test
fun resizeWindow_compact() {
onDevice().setDisplaySize(
widthSizeClass = WidthSizeClass.COMPACT,
heightSizeClass = HeightSizeClass.COMPACT
)
// Verify visual attributes or state restoration.
}
}
当您使用setDisplaySize()
调整显示尺寸时,不会影响设备的密度,因此,如果尺寸不适合目标设备,则测试将失败并出现UnsupportedDeviceOperationException
。为了防止在这种情况下运行测试,请使用RequiresDisplay
注解将其过滤掉。
@RunWith(AndroidJUnit4::class)
class ResizeDisplayTest {
@get:Rule(order = 1) var activityScenarioRule = activityScenarioRule<MainActivity>()
// Test rule for restoring device to its starting display size when a test case finishes.
@get:Rule(order = 2) var displaySizeRule: DisplaySizeRule = DisplaySizeRule()
/**
* Setting the display size to EXPANDED would fail in small devices, so the [RequiresDisplay]
* annotation prevents this test from being run on devices outside the EXPANDED buckets.
*/
@RequiresDisplay(
widthSizeClass = WidthSizeClassEnum.EXPANDED,
heightSizeClass = HeightSizeClassEnum.EXPANDED
)
@Test
fun resizeWindow_expanded() {
onDevice().setDisplaySize(
widthSizeClass = WidthSizeClass.EXPANDED,
heightSizeClass = HeightSizeClass.EXPANDED
)
// Verify visual attributes or state restoration.
}
}
StateRestorationTester
StateRestorationTester
类用于测试可组合组件的状态恢复,无需重新创建活动。这使得测试更快、更可靠,因为活动重新创建是一个涉及多个同步机制的复杂过程。
@Test
fun compactDevice_selectedEmailEmailRetained_afterConfigChange() {
val stateRestorationTester = StateRestorationTester(composeTestRule)
// Set content through the StateRestorationTester object.
stateRestorationTester.setContent {
MyApp()
}
// Simulate a config change.
stateRestorationTester.emulateSavedInstanceStateRestore()
}
窗口测试库
窗口测试库包含一些实用程序,可以帮助您编写依赖于或验证与窗口管理相关的功能(例如活动嵌入或可折叠功能)的测试。该构件可通过Google 的 Maven 存储库获得。
例如,您可以使用FoldingFeature()
函数生成自定义的FoldingFeature
,您可以在 Compose 预览中使用它。在 Java 中,使用createFoldingFeature()
函数。
在 Compose 预览中,您可以按以下方式实现FoldingFeature
@Preview(showBackground = true, widthDp = 480, heightDp = 480)
@Composable private fun FoldablePreview() =
MyApplicationTheme {
ExampleScreen(
displayFeatures = listOf(FoldingFeature(Rect(0, 240, 480, 240)))
)
}
此外,您可以使用TestWindowLayoutInfo()
函数在 UI 测试中模拟显示功能。以下示例模拟了一个在屏幕中心具有HALF_OPENED
垂直铰链的FoldingFeature
,然后检查布局是否符合预期。
Compose
import androidx.window.layout.FoldingFeature.Orientation.Companion.VERTICAL
import androidx.window.layout.FoldingFeature.State.Companion.HALF_OPENED
import androidx.window.testing.layout.FoldingFeature
import androidx.window.testing.layout.TestWindowLayoutInfo
import androidx.window.testing.layout.WindowLayoutInfoPublisherRule
@RunWith(AndroidJUnit4::class)
class MediaControlsFoldingFeatureTest {
@get:Rule(order=1)
val composeTestRule = createAndroidComposeRule<ComponentActivity>()
@get:Rule(order=2)
val windowLayoutInfoPublisherRule = WindowLayoutInfoPublisherRule()
@Test
fun foldedWithHinge_foldableUiDisplayed() {
composeTestRule.setContent {
MediaPlayerScreen()
}
val hinge = FoldingFeature(
activity = composeTestRule.activity,
state = HALF_OPENED,
orientation = VERTICAL,
size = 2
)
val expected = TestWindowLayoutInfo(listOf(hinge))
windowLayoutInfoPublisherRule.overrideWindowLayoutInfo(expected)
composeTestRule.waitForIdle()
// Verify that the folding feature is detected and media controls shown.
composeTestRule.onNodeWithTag("MEDIA_CONTROLS").assertExists()
}
}
视图
import androidx.window.layout.FoldingFeature.Orientation
import androidx.window.layout.FoldingFeature.State
import androidx.window.testing.layout.FoldingFeature
import androidx.window.testing.layout.TestWindowLayoutInfo
import androidx.window.testing.layout.WindowLayoutInfoPublisherRule
@RunWith(AndroidJUnit4::class)
class MediaControlsFoldingFeatureTest {
@get:Rule(order=1)
val activityRule = ActivityScenarioRule(MediaPlayerActivity::class.java)
@get:Rule(order=2)
val windowLayoutInfoPublisherRule = WindowLayoutInfoPublisherRule()
@Test
fun foldedWithHinge_foldableUiDisplayed() {
activityRule.scenario.onActivity { activity ->
val feature = FoldingFeature(
activity = activity,
state = State.HALF_OPENED,
orientation = Orientation.VERTICAL)
val expected = TestWindowLayoutInfo(listOf(feature))
windowLayoutInfoPublisherRule.overrideWindowLayoutInfo(expected)
}
// Verify that the folding feature is detected and media controls shown.
onView(withId(R.id.media_controls)).check(matches(isDisplayed()))
}
}
您可以在WindowManager 项目中找到更多示例。
其他资源
文档
示例
- WindowManager 示例
- Espresso Device 示例
- 现在在 Android 中
- 使用屏幕截图测试验证不同的屏幕尺寸
Codelabs