为了使您的通知在不同版本的 Android 上看起来最佳,请使用标准通知模板来构建您的通知。如果您想在通知中提供更多内容,请考虑使用可扩展通知模板之一。
但是,如果系统模板不能满足您的需求,您可以使用您自己的通知布局。
为内容区域创建自定义布局
如果您需要自定义布局,可以将NotificationCompat.DecoratedCustomViewStyle
应用于您的通知。此 API 允许您为通常由标题和文本内容占据的内容区域提供自定义布局,同时仍使用系统装饰来显示通知图标、时间戳、副文本和操作按钮。
此 API 与可扩展通知模板的工作原理类似,其构建方式如下
- 使用
NotificationCompat.Builder
构建基本通知。 - 调用
setStyle()
,并向其传递NotificationCompat.DecoratedCustomViewStyle
的实例。 - 将您的自定义布局膨胀为
RemoteViews
的实例。 - 调用
setCustomContentView()
以设置折叠通知的布局。 - 可选地,还可以调用
setCustomBigContentView()
以设置扩展通知的不同布局。
准备布局
您需要一个small
和large
布局。对于此示例,small
布局可能如下所示
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="Small notification, showing only a title" />
</LinearLayout>
而large
布局可能如下所示
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="300dp"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Large notification, showing a title and a body." />
<TextView
android:id="@+id/notification_body"
style="@style/TextAppearance.Compat.Notification.Line2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="This is the body. The height is manually forced to 300dp." />
</LinearLayout>
构建并显示通知
布局准备就绪后,您可以按以下示例所示使用它们
Kotlin
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager // Get the layouts to use in the custom notification. val notificationLayout = RemoteViews(packageName, R.layout.notification_small) val notificationLayoutExpanded = RemoteViews(packageName, R.layout.notification_large) // Apply the layouts to the notification. val customNotification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build() notificationManager.notify(666, customNotification)
Java
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); // Get the layouts to use in the custom notification RemoteViews notificationLayout = new RemoteViews(getPackageName(), R.layout.notification_small); RemoteViews notificationLayoutExpanded = new RemoteViews(getPackageName(), R.layout.notification_large); // Apply the layouts to the notification. Notification customNotification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(new NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build(); notificationManager.notify(666, customNotification);
请注意,通知的背景颜色可能因设备和版本而异。在您的自定义布局中,为文本应用 Support Library 样式(如TextAppearance_Compat_Notification
)和标题应用TextAppearance_Compat_Notification_Title
,如下例所示。这些样式会适应颜色变化,因此您最终不会出现黑底黑字或白底白字。
<TextView android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_weight="1" android:text="@string/notification_title" android:id="@+id/notification_title" style="@style/TextAppearance.Compat.Notification.Title" />
避免在RemoteViews
对象上设置背景图像,因为您的文本可能变得难以阅读。
当您在用户使用应用时触发通知时,结果类似于图 1
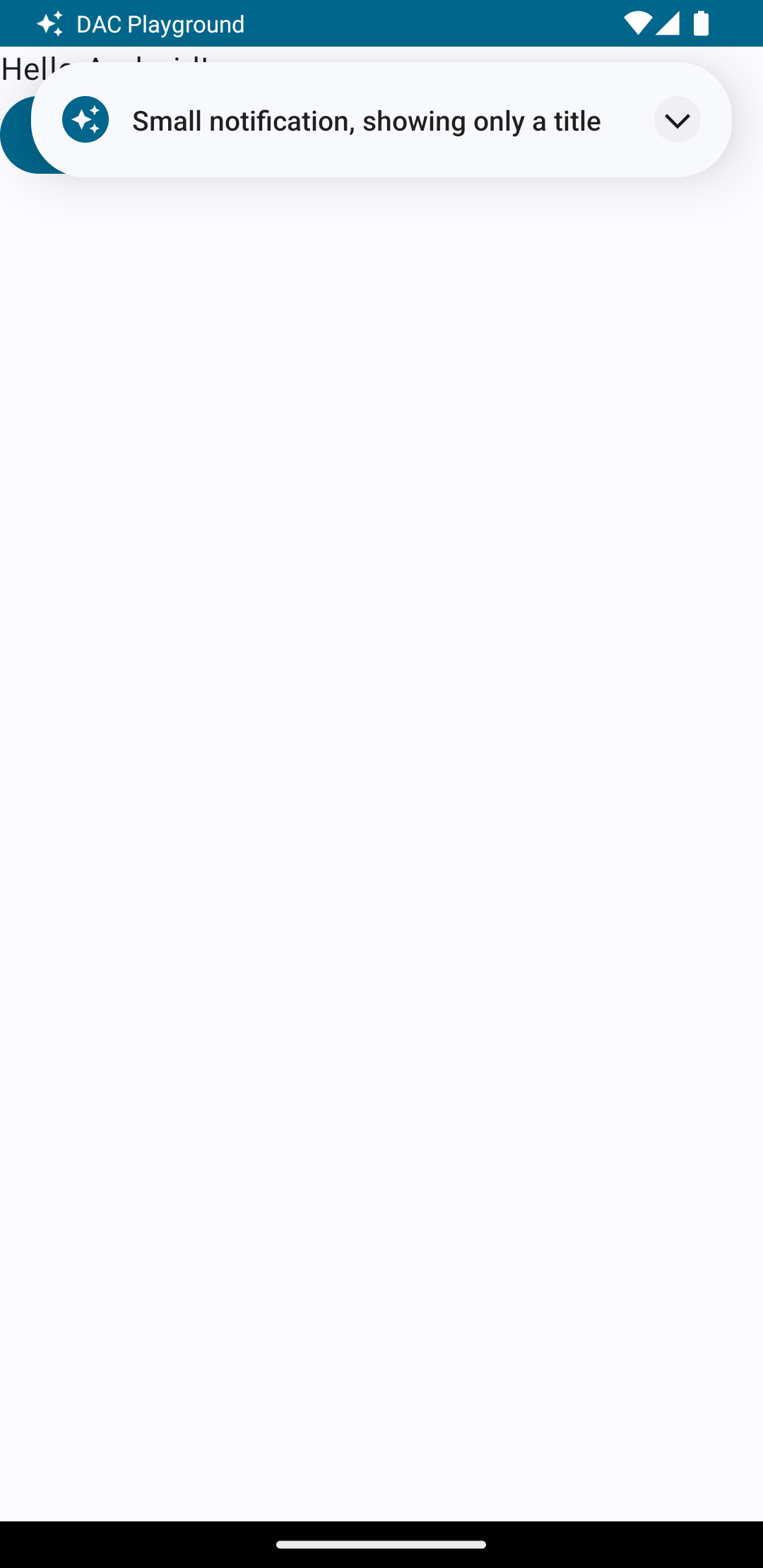
点击展开箭头会展开通知,如图 2 所示
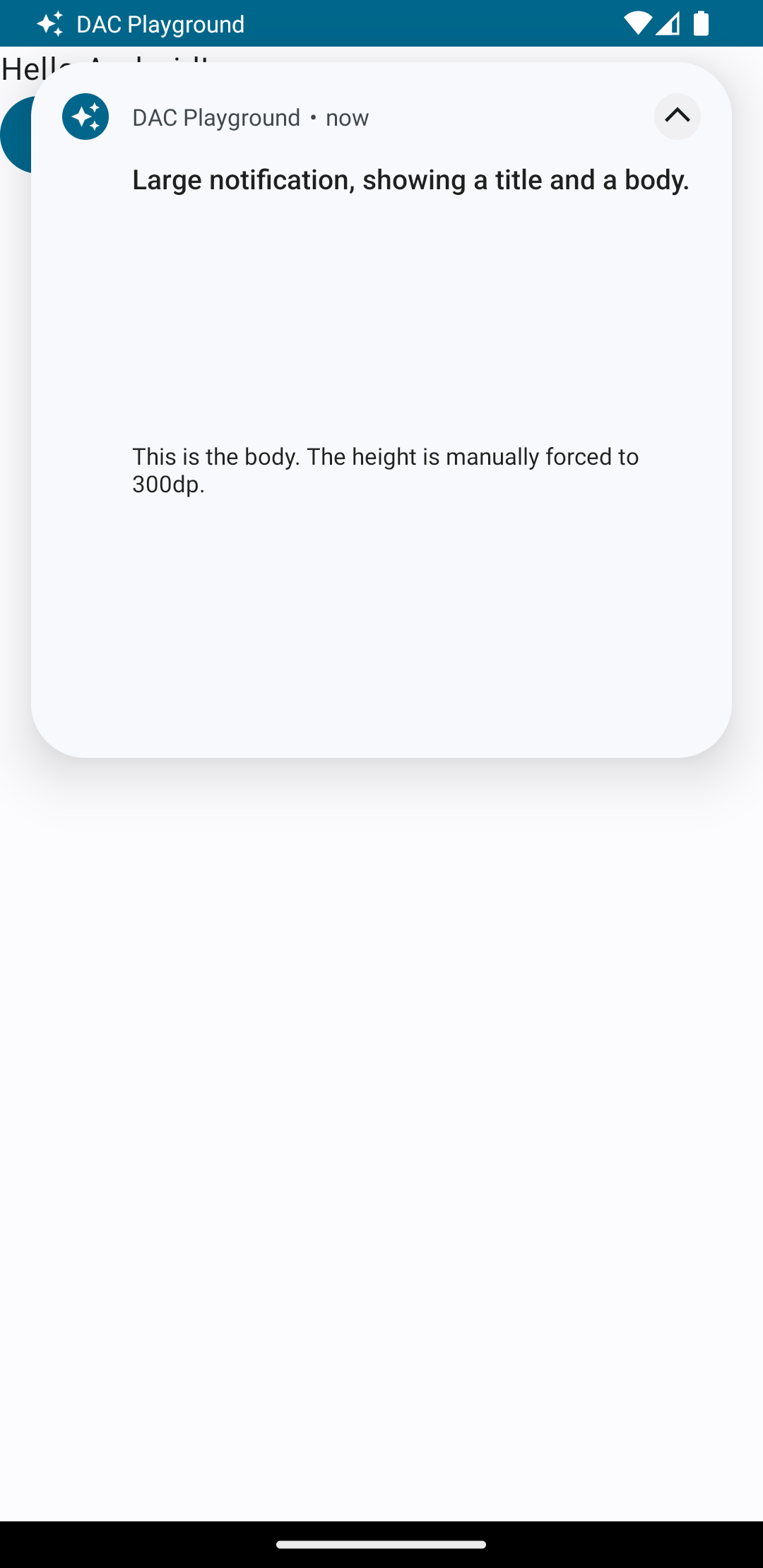
通知超时时间结束后,通知仅在系统栏中可见,这看起来像图 3
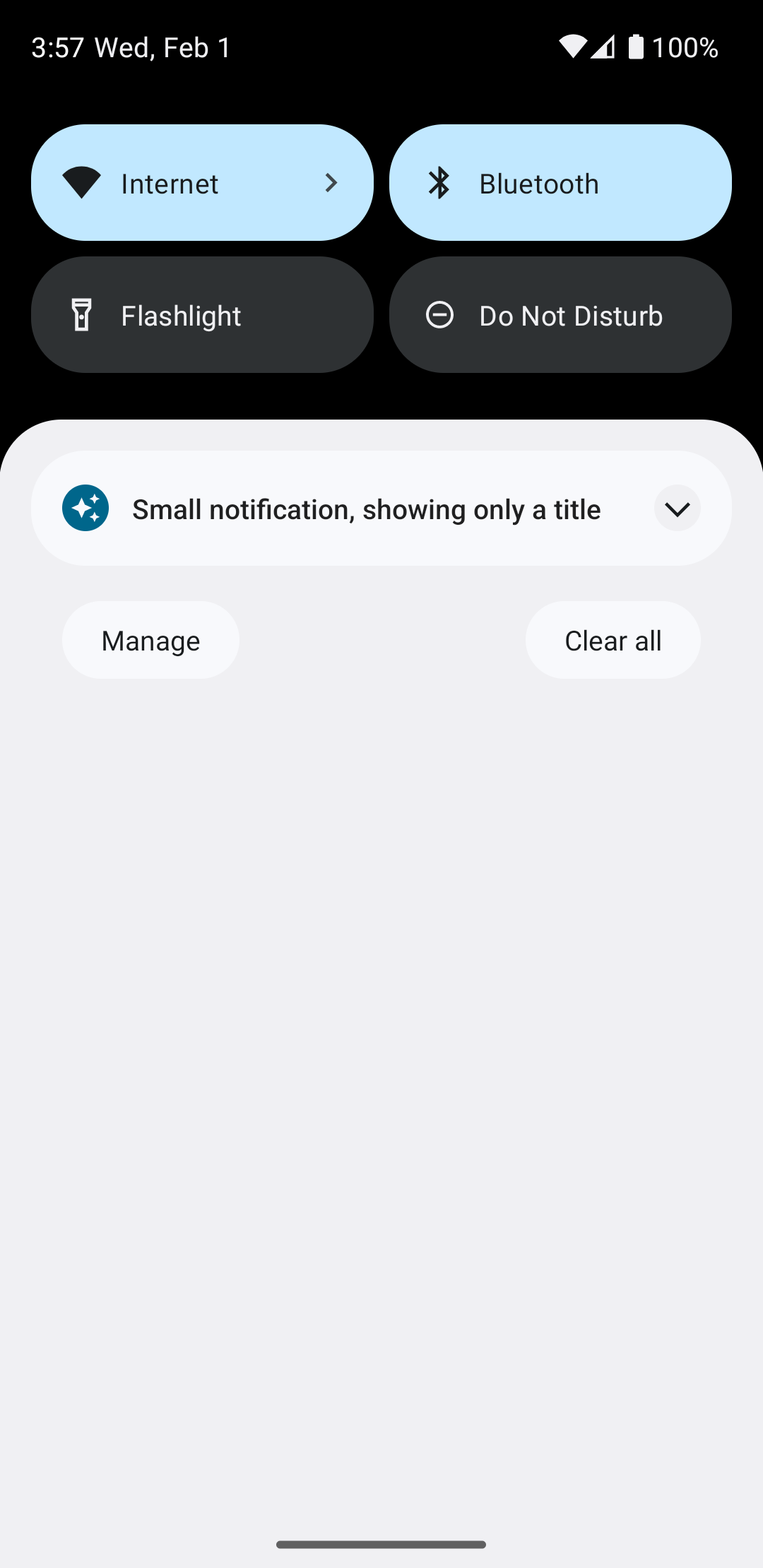
点击展开箭头会展开通知,如图 4 所示
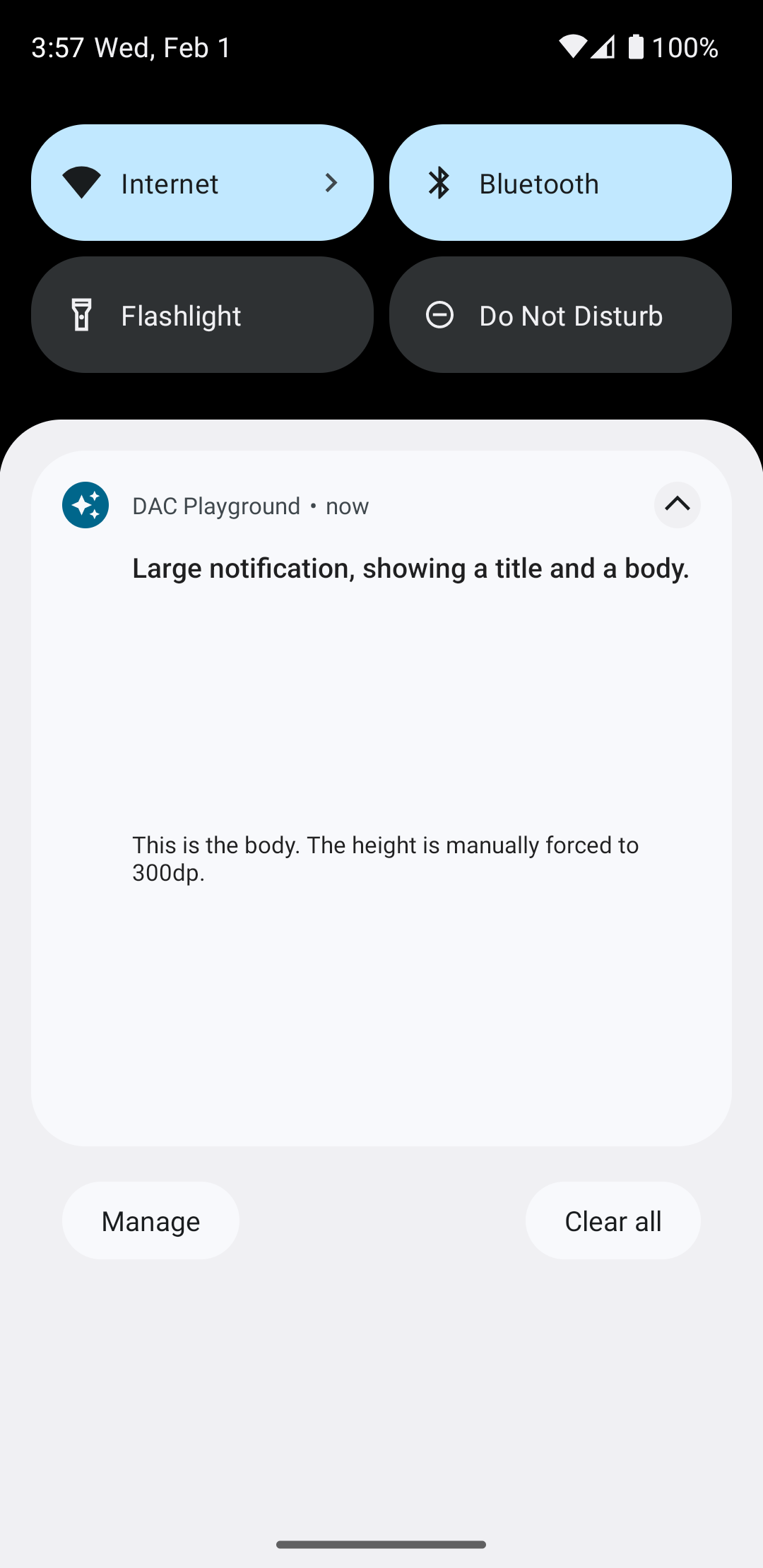
创建完全自定义的通知布局
如果您不希望您的通知以标准通知图标和标题进行装饰,请按照上述步骤操作,但不要调用setStyle()
。