在 Android 12.0(API 级别 31)及更高版本中,系统提供 CallStyle
通知模板来区分呼叫通知和其他类型的通知。使用此模板创建传入或正在进行的呼叫通知。该模板支持包含呼叫者信息和必需操作(例如接听或拒绝呼叫)的大格式通知。
由于传入和正在进行的呼叫是高优先级事件,因此这些通知在通知栏中获得最高优先级。此排名还使系统能够将这些优先呼叫转发到其他设备。
该 CallStyle
通知模板包含以下必需操作
- 接听 或 拒绝 用于传入呼叫。
- 挂断 用于正在进行的呼叫。
- 接听 或 挂断 用于呼叫筛选。
此样式中的操作显示为按钮,系统会自动添加相应的图标和文本。不支持手动标记按钮。有关通知设计原则的更多信息,请参阅 通知。
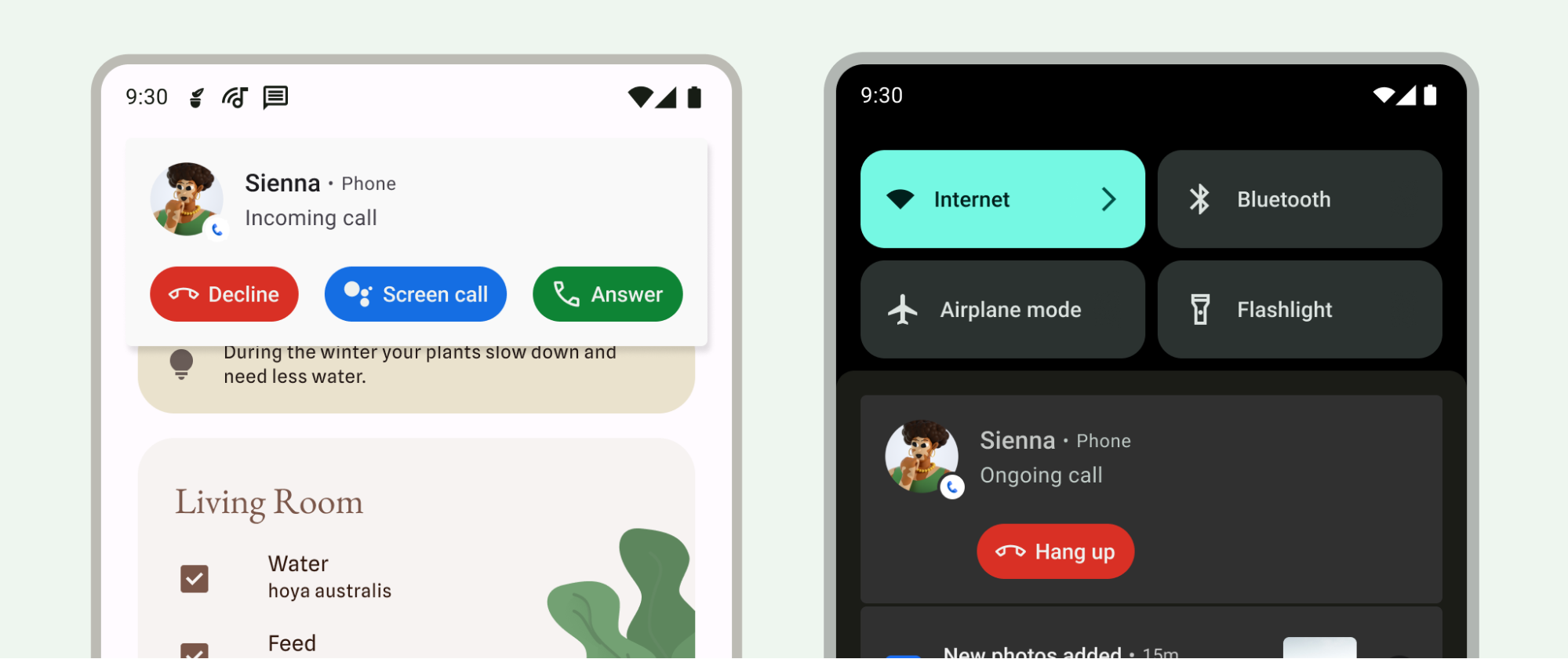
必需操作作为意图传递,例如 hangupIntent
和 answerIntent
在以下部分中。它们每个都是对系统维护的令牌的引用。令牌是一个轻量级对象,可以在不同的应用和进程之间传递。系统负责管理令牌的生命周期并确保 PendingIntent
可用,即使创建它的应用不再运行也是如此。当您向另一个应用提供 PendingIntent
时,您是在授予它执行指定操作的权限,例如拒绝或接听。即使创建意图的应用当前未运行,也会授予此权限。有关更多信息,请参阅 PendingIntent
的参考文档。
从 Android 14(API 级别 34)开始,您可以将呼叫通知配置为不可取消。为此,请使用 CallStyle
通知以及 Notification.FLAG_ONGOING_EVENT
通过 Notification.Builder#setOngoing(true)
。
以下是使用 CallStyle
通知中各种方法的示例。
Kotlin
// Create a new call, setting the user as the caller. val incomingCaller = Person.Builder() .setName("Jane Doe") .setImportant(true) .build()
Java
// Create a new call with the user as the caller. Person incomingCaller = new Person.Builder() .setName("Jane Doe") .setImportant(true) .build();
传入呼叫
使用 forIncomingCall()
方法为传入呼叫创建呼叫样式通知。
Kotlin
// Create a call style notification for an incoming call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller)
Java
// Create a call style notification for an incoming call. Notification.Builder builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller);
正在进行的呼叫
使用 forOngoingCall()
方法为正在进行的呼叫创建呼叫样式通知。
Kotlin
// Create a call style notification for an ongoing call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller)
Java
// Create a call style notification for an ongoing call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller);
筛选呼叫
使用 forScreeningCall()
方法为筛选呼叫创建呼叫样式通知。
Kotlin
// Create a call style notification for screening a call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller)
Java
// Create a call style notification for screening a call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller);
提供跨更多 Android 版本的兼容性
将 CallStyle
通知与 API 版本 30 或更早版本关联的前台服务,以便为它们分配在 API 级别 31 或更高版本中获得的高排名。此外,CallStyle
API 版本 30 或更早版本上的通知可以通过将通知标记为彩色来实现类似的排名,使用 setColorized()
方法。
将 Telecom API 与 CallStyle
通知一起使用。有关更多信息,请参阅 Telecom 框架概述。