简介
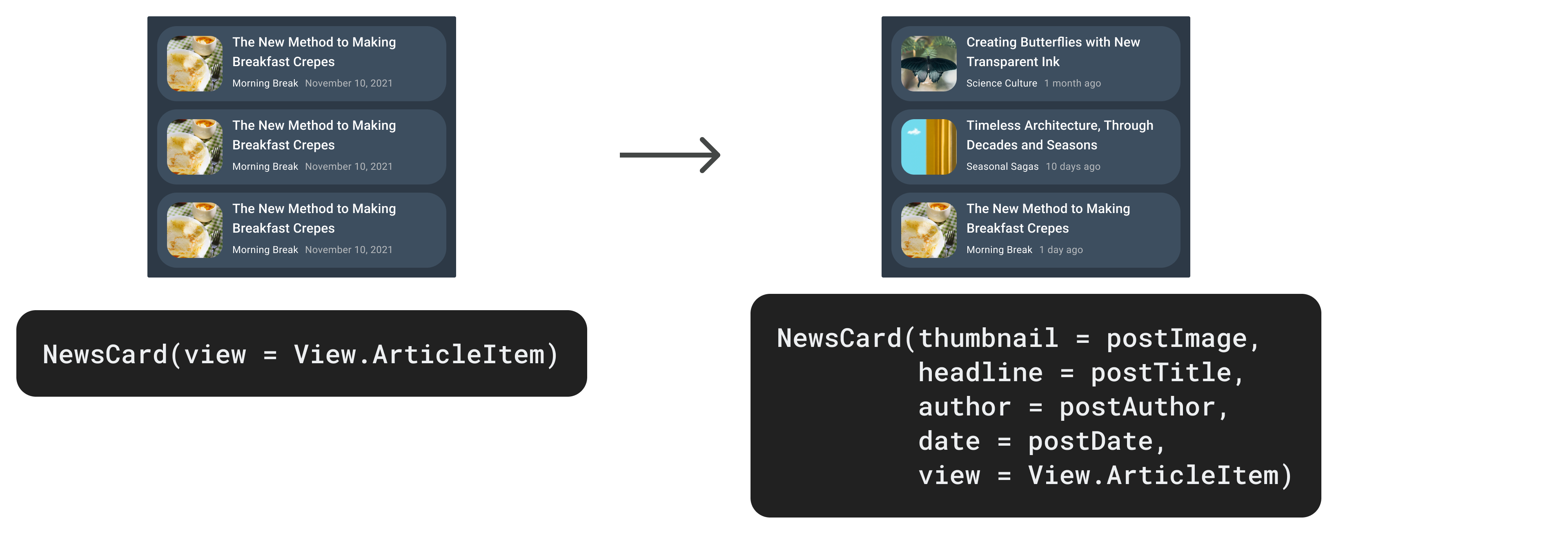
大多数 UI 设计的内容并非静态的——它们会根据数据而变化。在本节中,我们将通过内容参数向设计添加数据,这允许设计师指定设计中的哪个部分应填充数据。
在 Figma 中添加参数
让我们向组件添加内容参数。
- 切换到 Figma。在 NewsCardTutorial 中,选择**hero-item 变体**中的**缩略图图像**图层(Mac 上按⌘ + 点击,Windows 和 Linux 上按 Ctrl + 点击图像)。
在 Relay for Figma 插件中,点击**+**并从下拉菜单中选择
image-content
,然后将名称更改为“thumbnail”。选择**标题文本**图层,点击 + 并选择
text-content
。对 hero-item 变体中的**作者**和**日期**文本图层重复相同的步骤。分别将其命名为“headline”、“author”和“date”。点击插件中的缩略图参数。请注意,在每个组件变体中,缩略图图层都是图像且已被选中。
由于这三个图层具有相同的名称(“thumbnail”)并且是相同的数据类型(image-content),因此内容参数已连接到所有三个变体中。这意味着一个参数会向多个变体提供相同的数据。标题、作者和日期参数也是如此。
保存命名版本
现在让我们将此版本标记为已准备好导入到代码中。
打开 Figma Relay 插件(如果尚未打开)。
点击**与开发者共享**。
在**与开发者共享**屏幕中,输入版本的名称和描述。
**示例标题**: 添加了参数
**示例描述**: 向卡片添加了内容参数
点击**保存**。
在 Android Studio 中更新组件
让我们更新**NewsCard**组件
在 Android Studio 中,确保“项目”工具窗口处于**Android 视图**。然后右键点击
app/ui-packages/news_card/
,然后点击**更新 UI 包**。点击
来构建您的项目。这将采用更新的 UI 包并生成组合代码的更新版本。
如果您查看
app/java (generated)/com/example/hellonews/newscard/NewsCard.kt
,您会看到我们添加的内容参数(thumbnail
、headline
、author
、date
)出现在我们组合的组件参数列表中。// View to select for NewsCard enum class View { HeroItem, ArticleItem, AudioItem } /** * News card component intended to display news items for a list * * This composable was generated from the UI package 'news_card'. * Generated code; don't edit directly. */ @Composable fun NewsCard( modifier: Modifier = Modifier, view: View = View.HeroItem, thumbnail: Painter = EmptyPainter(), headline: String = "", author: String = "", date: String = "" ) { ...
集成到应用中
让我们回顾一下我们的应用,它的 UI 我们还没有修改。它包含常规新闻报道列表和音频报道列表。我们需要将我们的**NewsCard**组件添加到这两个组合组件中。
- **PostListArticleStories**组合组件负责常规新闻报道。
- **postTop**参数表示头条新闻。
- **posts**参数表示其余的新闻。
- **PostListAudioStories**组合组件呈现音频新闻报道。
在
app/java/com/example/hellonews/ui/home/HomeScreen.kt
中,在文件顶部的其他导入行旁边添加以下导入:importcom.example.hellonews.newscard.NewsCard
import com.example.hellonews.newscard.View
仍在**HomeScreen.kt**中,向下滚动到**PostListArticleStories**。
@Composable fun HomeScreen(...) @Composable private fun PostList(...) @Composable private fun PostListArticleStoriesSection(...) @Composable private fun SearchArticlesSection(...) @Composable private fun PostListArticleStories( postTop: Post, posts: List<Post>, createOnTapped: (String, String) -> () -> Unit ) {...} @Composable private fun AudioStoriesTitle(...) @Composable private fun PostListAudioStories(...) @Composable fun Dialog(...) ...
对于**postTop**,使用**HeroItem**视图将**Text**组件替换为我们新导入的**NewsCard**。
@Composable private fun PostListArticleStories( postTop: Post, posts: List<Post>, createOnTapped: (String, String) -> () -> Unit ) { ... Column( horizontalAlignment = Alignment.Start, modifier = ... ) { Spacer(modifier = Modifier.size(12.dp)) NewsCard( thumbnail = painterResource(postTop.imageId), headline = postTop.title, author = postTop.metadata.author.name, date = postTop.metadata.date, view = View.HeroItem ) Spacer(modifier = Modifier.size(12.dp)) ... } }
对于每个**post**,使用**ArticleItem**视图将**Text**组件替换为我们新导入的**NewsCard**。
@Composable private fun PostListArticleStories( postTop: Post, posts: List<Post>, createOnTapped: (String, String) -> () -> Unit ) { ... Column( horizontalAlignment = Alignment.Start, modifier = ... ) { ... posts.forEach { post -> NewsCard( thumbnail = painterResource(post.imageId), headline = post.title, author = post.metadata.author.name, date = post.metadata.date, view = View.ArticleItem ) Spacer(modifier = Modifier.size(12.dp)) } } }
我们可以对底部的音频报道做同样的事情。仍在
HomeScreen.kt
中,向下滚动到**PostListAudioStories**,大约在第 260 行。@Composable fun HomeScreen(...) @Composable private fun PostList(...) @Composable private fun PostListArticleStoriesSection(...) @Composable private fun SearchArticlesSection(...) @Composable private fun PostListArticleStories(...) @Composable private fun AudioStoriesTitle(...) @Composable private fun PostListAudioStories( posts: List<Post>, createOnTapped: (String, String) -> () -> Unit ) {...} @Composable fun Dialog(...) ...
对于每个post,使用AudioItem视图将**Text**组件替换为我们新导入的**NewsCard**。
@Composable private fun PostListAudioStories( posts: List<Post>, createOnTapped: (String, String) -> () -> Unit ) { Column( horizontalAlignment = ..., modifier = ... ) { posts.forEach { post -> NewsCard( thumbnail = painterResource(post.imageId), headline = post.title, author = post.metadata.author.name, date = post.metadata.date, view = View.AudioItem ) Spacer(modifier = Modifier.size(12.dp)) } } }
点击
再次构建您的项目,并在预览(分屏视图)中查看结果。
下一步
接下来,我们将向我们的应用添加一些交互。
为您推荐
- 注意:当 JavaScript 关闭时,链接文本会显示。
- 向设计添加交互处理程序
- 处理设计变体
- 在 Android Studio 中将设计转换为代码