使用 Compose,您可以创建由多边形组成的形状。例如,您可以创建以下类型的形状
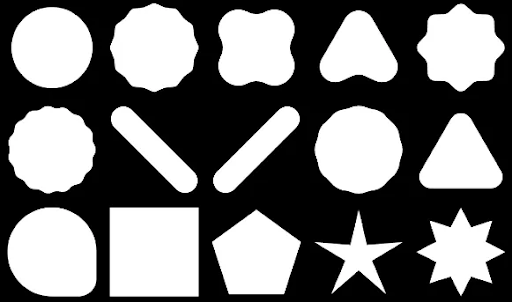
要在 Compose 中创建自定义圆角多边形,请将 graphics-shapes
依赖项添加到您的 app/build.gradle
中
implementation "androidx.graphics:graphics-shapes:1.0.0-rc01"
此库允许您创建由多边形组成的形状。虽然多边形形状只有直边和锐角,但这些形状允许可选的圆角。它简化了两种不同形状之间的变形。任意形状之间的变形很困难,并且往往是设计时的问题。但此库通过在具有相似多边形结构的这些形状之间变形使其变得简单。
创建多边形
以下代码片段在绘图区域的中心创建了一个具有 6 个点的基本多边形形状
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 6, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2 ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Blue) } } .fillMaxSize() )
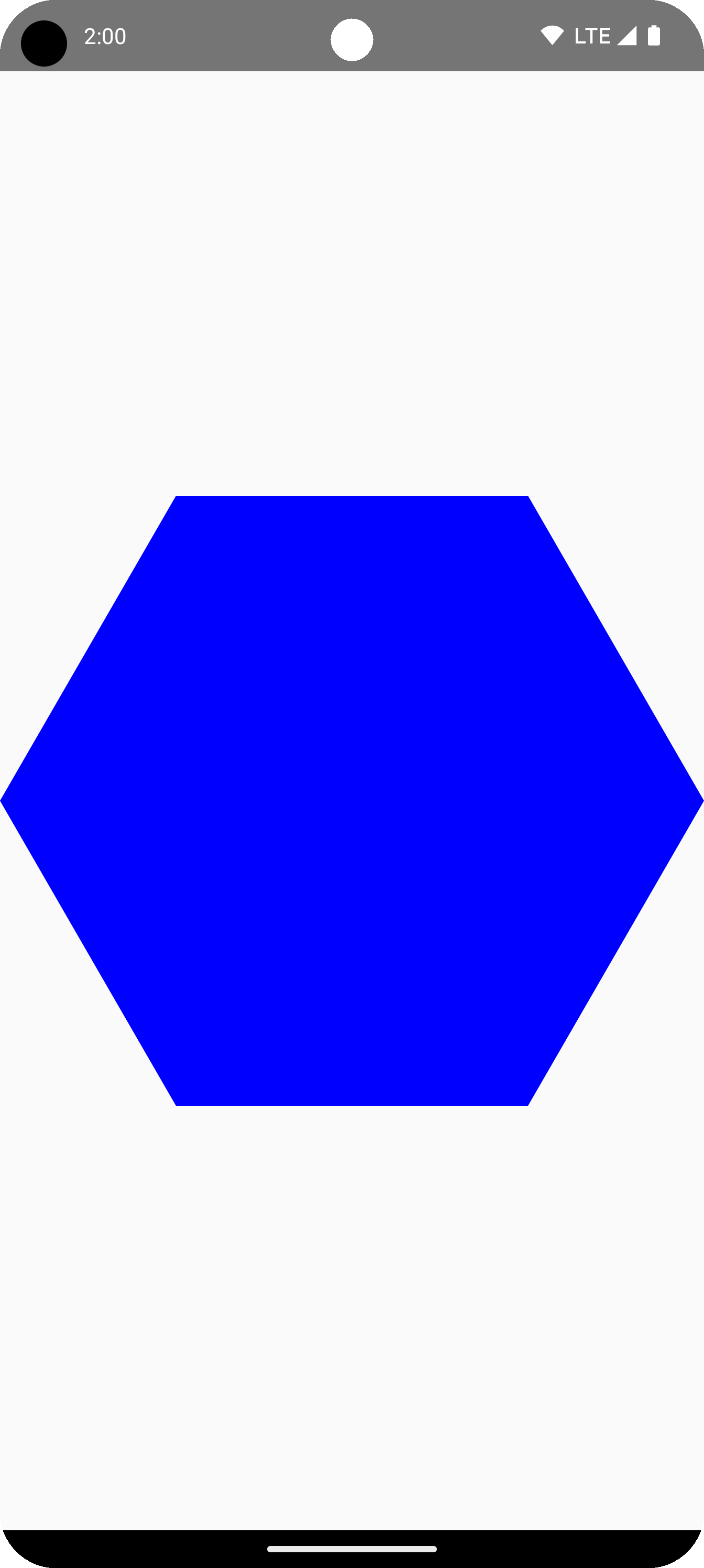
在此示例中,库创建了一个 RoundedPolygon
,它保存表示请求形状的几何图形。为了在 Compose 应用中绘制该形状,您必须从中获取一个 Path
对象,以将形状转换为 Compose 知道如何绘制的形式。
圆角多边形
要圆角多边形,请使用 CornerRounding
参数。这需要两个参数,radius
和 smoothing
。每个圆角由 1-3 个三次曲线组成,其中心具有圆弧形状,而两个侧边(“侧翼”)曲线从形状的边缘过渡到中心曲线。
半径
radius
是用于圆角顶点的圆的半径。
例如,以下圆角三角形如下所示
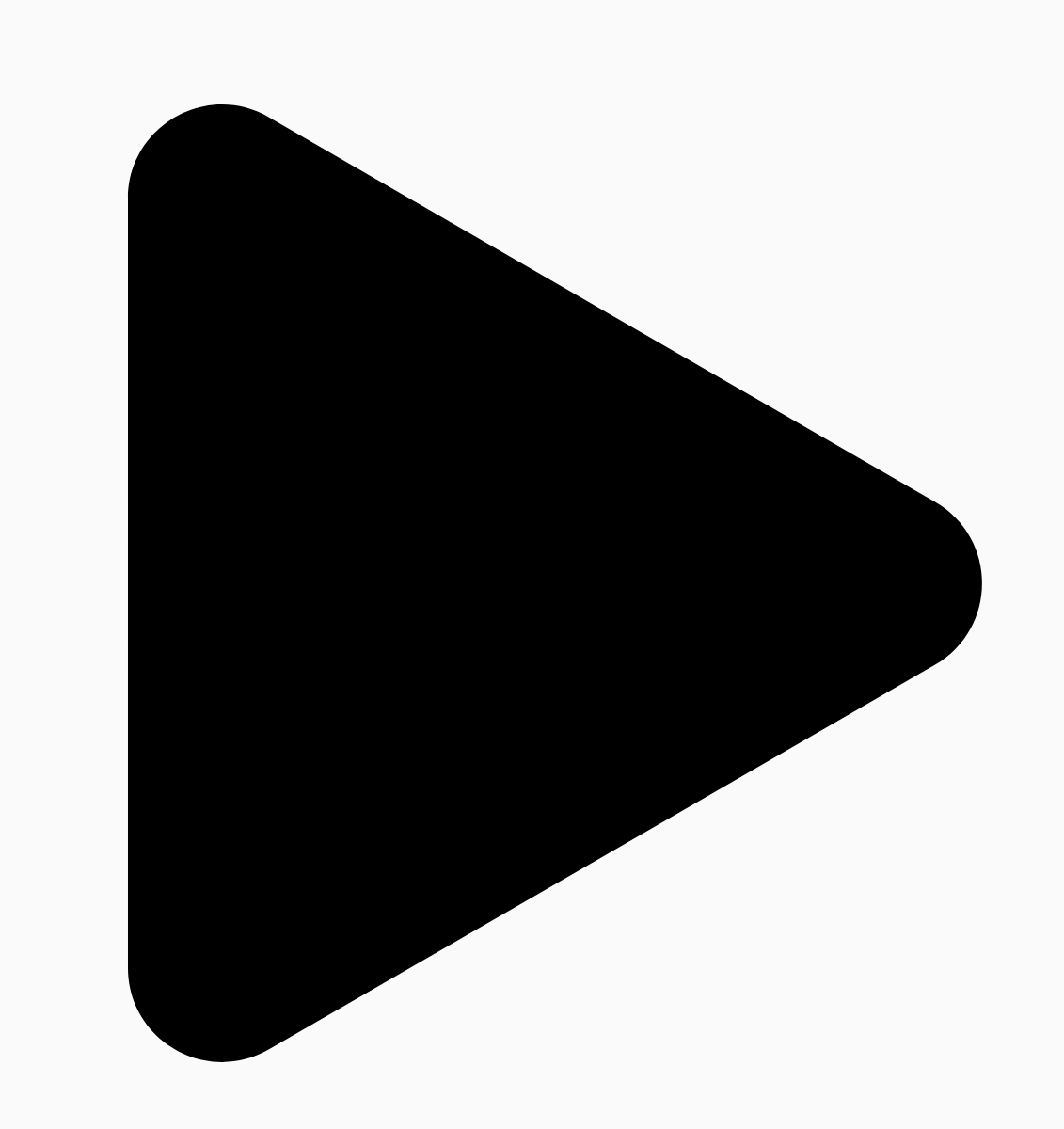
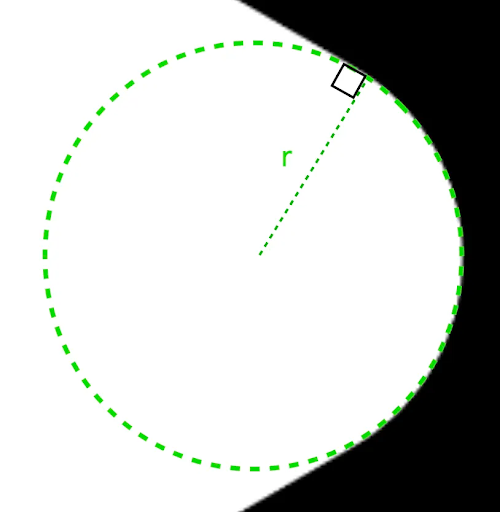
r
决定圆角的圆形圆角大小。平滑度
平滑度是一个因子,它决定了从角的圆形圆角部分到边缘需要多长时间。0 的平滑度因子(未平滑,CornerRounding
的默认值)会导致纯粹的圆形圆角。非零平滑度因子(最高 1.0)会导致角由三个单独的曲线圆角。
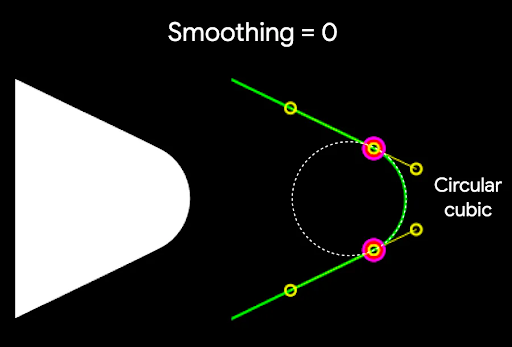
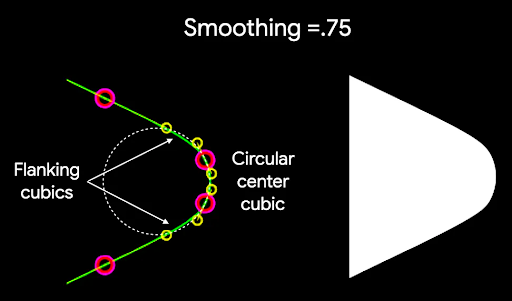
例如,下面的代码片段说明了将平滑度设置为 0 与 1 的细微差别
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Black) } } .size(100.dp) )
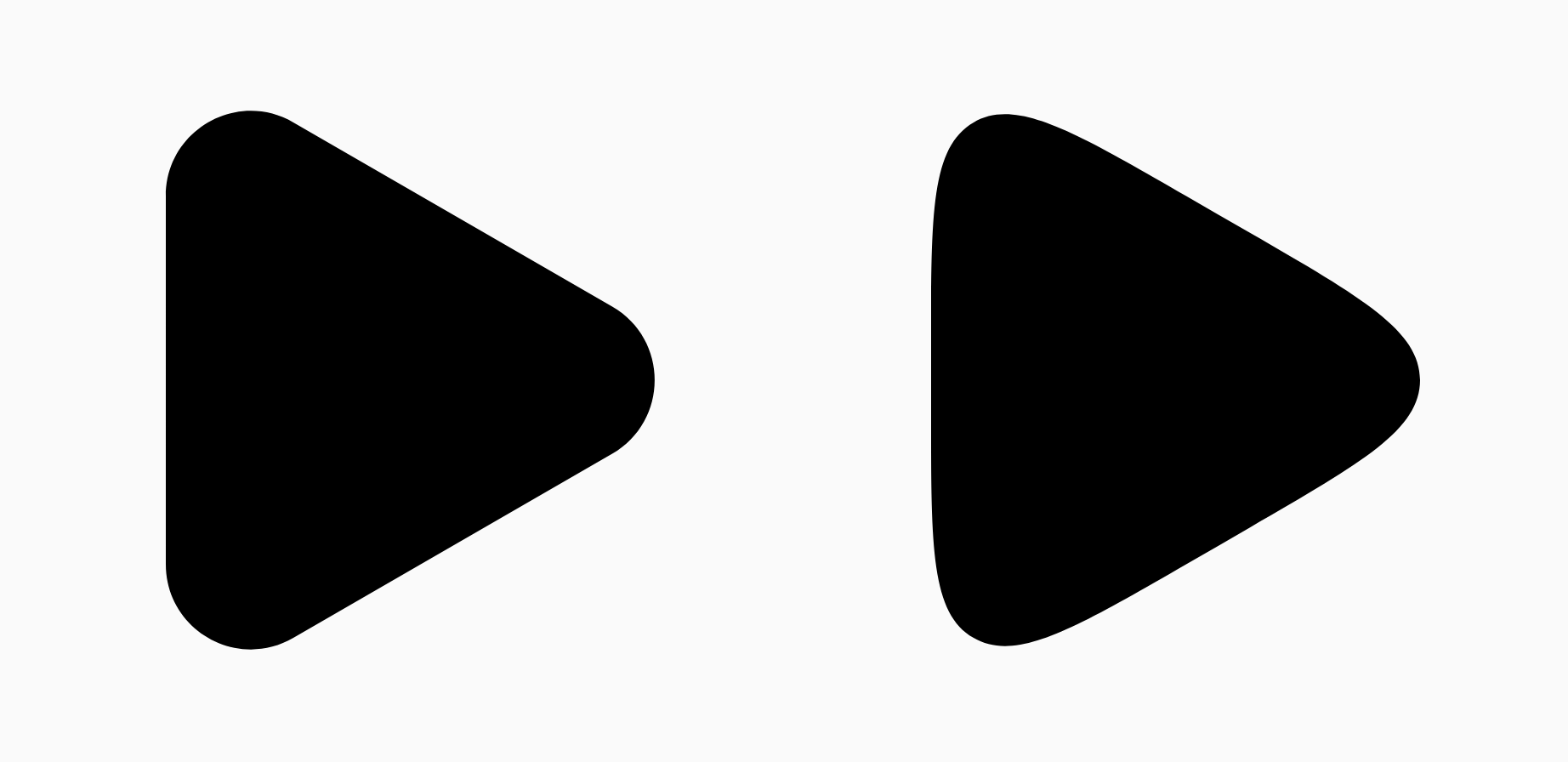
大小和位置
默认情况下,形状在中心(0, 0
)周围创建半径为 1
的形状。此半径表示中心与形状所基于的多边形的外部顶点之间的距离。请注意,圆角会导致形状变小,因为圆角将比被圆角的顶点更靠近中心。要调整多边形的大小,请调整 radius
值。要调整位置,请更改多边形的 centerX
或 centerY
。或者,使用标准 DrawScope
变换函数(例如 DrawScope#translate()
)变换对象以更改其大小、位置和旋转。
变形形状
Morph
对象是一个新的形状,表示两个多边形形状之间的动画。要在两个形状之间变形,请创建两个 RoundedPolygons
和一个 Morph
对象,该对象接受这两个形状。要计算开始形状和结束形状之间的形状,请提供一个介于零和一之间的 progress
值以确定其在开始(0)和结束(1)形状之间的形式
Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = 0.5f).asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
在上面的示例中,进度正好位于两个形状(圆角三角形和正方形)之间的一半,产生以下结果
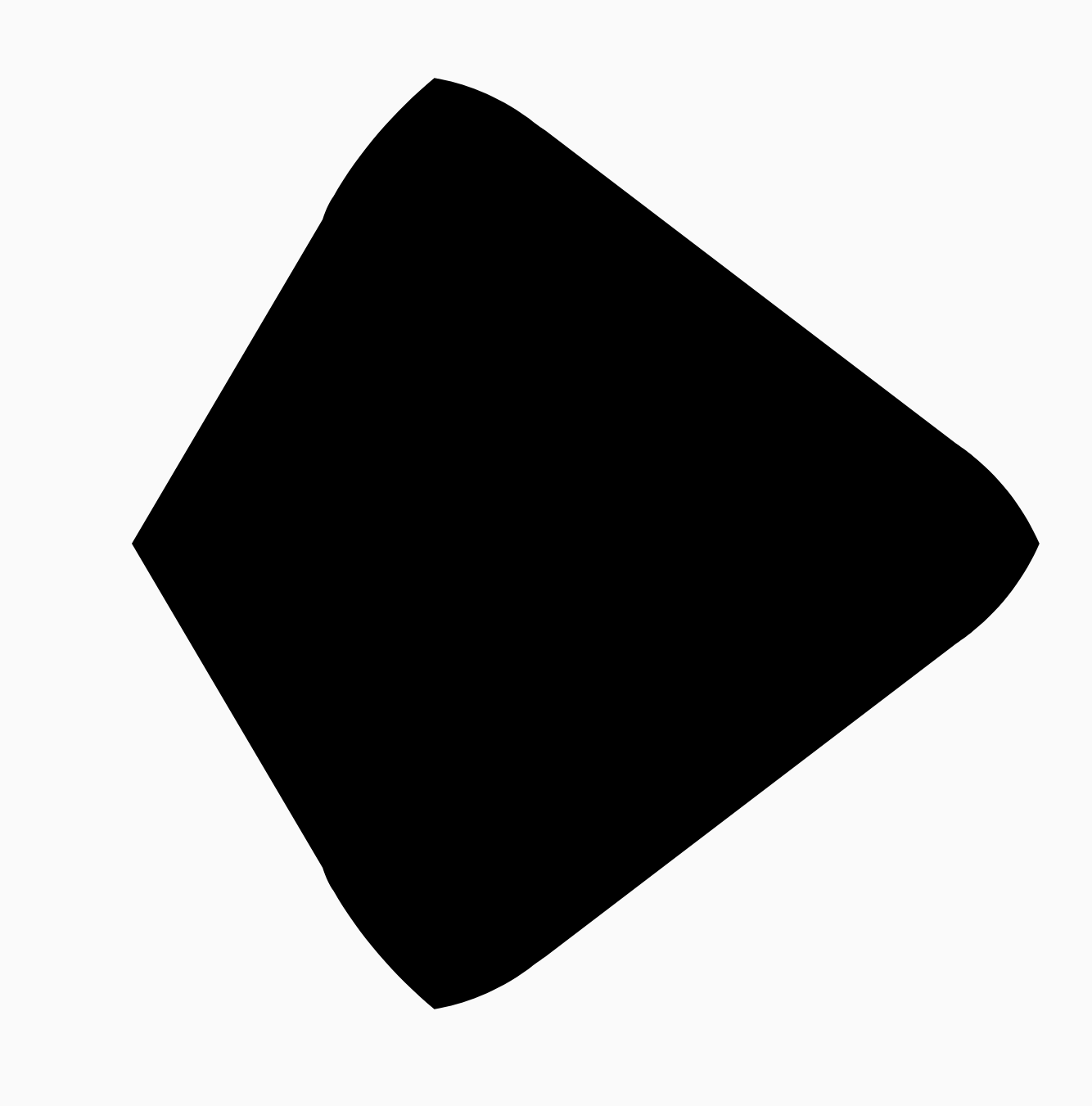
在大多数情况下,变形作为动画的一部分完成,而不仅仅是静态渲染。要在这两者之间进行动画处理,您可以使用 Compose 中的标准 动画 API 随时间推移更改进度值。例如,您可以无限期地在这两个形状之间进行变形动画,如下所示
val infiniteAnimation = rememberInfiniteTransition(label = "infinite animation") val morphProgress = infiniteAnimation.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(500), repeatMode = RepeatMode.Reverse ), label = "morph" ) Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = morphProgress.value) .asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
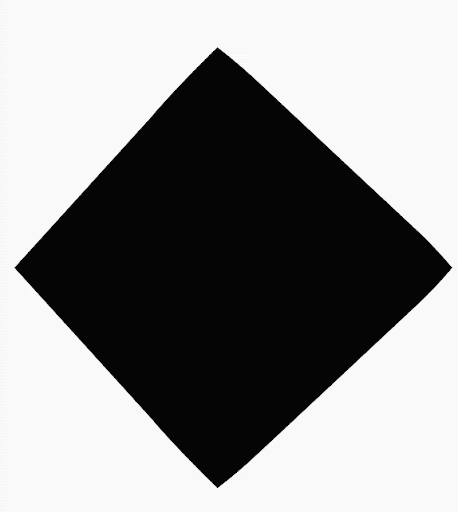
使用多边形作为剪辑
在 Compose 中使用 clip
修饰符更改组合项的渲染方式并利用围绕剪辑区域绘制的阴影是很常见的
fun RoundedPolygon.getBounds() = calculateBounds().let { Rect(it[0], it[1], it[2], it[3]) } class RoundedPolygonShape( private val polygon: RoundedPolygon, private var matrix: Matrix = Matrix() ) : Shape { private var path = Path() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { path.rewind() path = polygon.toPath().asComposePath() matrix.reset() val bounds = polygon.getBounds() val maxDimension = max(bounds.width, bounds.height) matrix.scale(size.width / maxDimension, size.height / maxDimension) matrix.translate(-bounds.left, -bounds.top) path.transform(matrix) return Outline.Generic(path) } }
然后,您可以使用多边形作为剪辑,如下面的代码片段所示
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier .clip(clip) .background(MaterialTheme.colorScheme.secondary) .size(200.dp) ) { Text( "Hello Compose", color = MaterialTheme.colorScheme.onSecondary, modifier = Modifier.align(Alignment.Center) ) }
这将导致以下结果
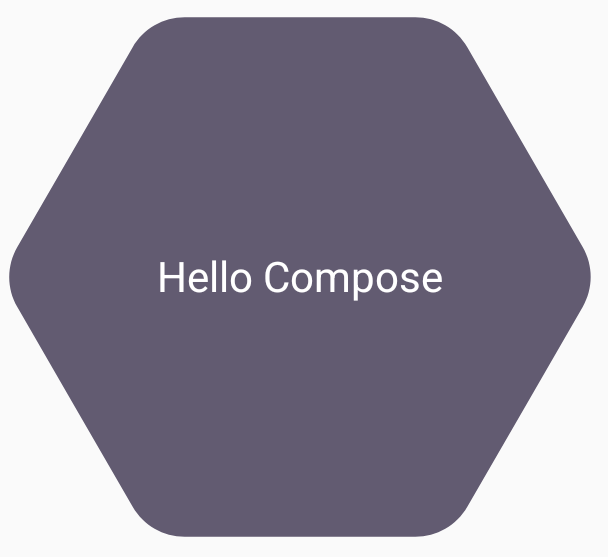
这可能与之前渲染的内容看起来没有什么不同,但它允许利用 Compose 中的其他功能。例如,此技术可用于剪辑图像并在剪辑区域周围应用阴影
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .graphicsLayer { this.shadowElevation = 6.dp.toPx() this.shape = clip this.clip = true this.ambientShadowColor = Color.Black this.spotShadowColor = Color.Black } .size(200.dp) ) }
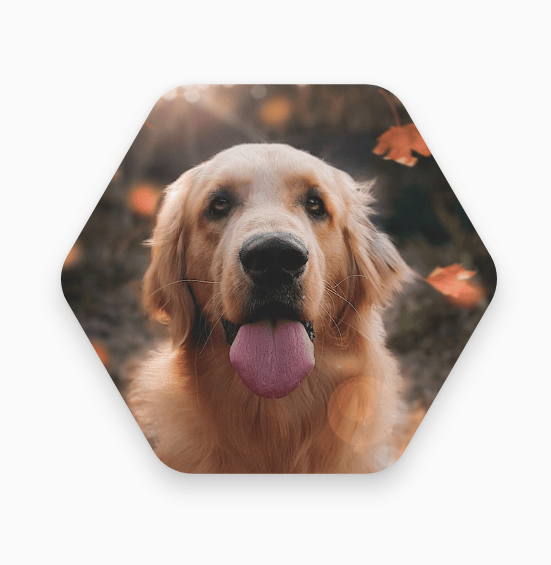
单击时变形按钮
您可以使用 graphics-shape
库创建一个在按下时在两个形状之间变形的按钮。首先,创建一个扩展 Shape
的 MorphPolygonShape
,并将其缩放和平移以适合。请注意传递进度,以便可以对形状进行动画处理
class MorphPolygonShape( private val morph: Morph, private val percentage: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } }
要使用此变形形状,请创建两个多边形,shapeA
和 shapeB
。创建并记住 Morph
。然后,将变形应用于按钮作为剪辑轮廓,使用按下时的 interactionSource
作为动画背后的驱动力
val shapeA = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 6, rounding = CornerRounding(0.1f) ) } val morph = remember { Morph(shapeA, shapeB) } val interactionSource = remember { MutableInteractionSource() } val isPressed by interactionSource.collectIsPressedAsState() val animatedProgress = animateFloatAsState( targetValue = if (isPressed) 1f else 0f, label = "progress", animationSpec = spring(dampingRatio = 0.4f, stiffness = Spring.StiffnessMedium) ) Box( modifier = Modifier .size(200.dp) .padding(8.dp) .clip(MorphPolygonShape(morph, animatedProgress.value)) .background(Color(0xFF80DEEA)) .size(200.dp) .clickable(interactionSource = interactionSource, indication = null) { } ) { Text("Hello", modifier = Modifier.align(Alignment.Center)) }
当点击框时,这将导致以下动画
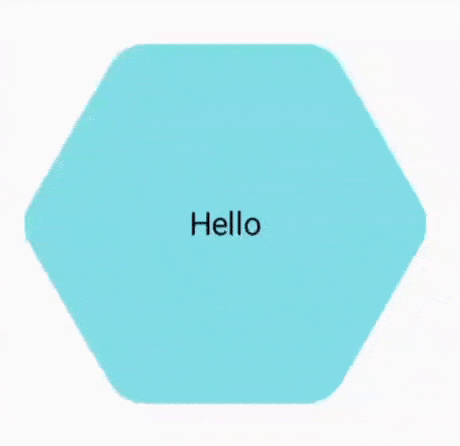
无限动画形状变形
要无限期地对变形形状进行动画处理,请使用 rememberInfiniteTransition
。下面是配置文件图片随时间无限期地更改形状(并旋转)的示例。此方法对上面显示的 MorphPolygonShape
进行了一些小的调整
class CustomRotatingMorphShape( private val morph: Morph, private val percentage: Float, private val rotation: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) matrix.rotateZ(rotation) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } } @Preview @Composable private fun RotatingScallopedProfilePic() { val shapeA = remember { RoundedPolygon( 12, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 12, rounding = CornerRounding(0.2f) ) } val morph = remember { Morph(shapeA, shapeB) } val infiniteTransition = rememberInfiniteTransition("infinite outline movement") val animatedProgress = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(2000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) val animatedRotation = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 360f, animationSpec = infiniteRepeatable( tween(6000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .clip( CustomRotatingMorphShape( morph, animatedProgress.value, animatedRotation.value ) ) .size(200.dp) ) } }
此代码给出了以下有趣的结果
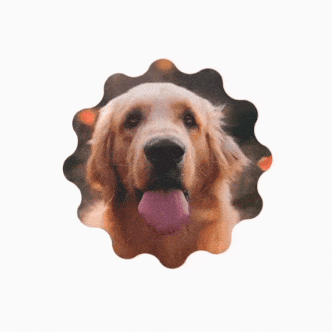
自定义多边形
如果由规则多边形创建的形状不能满足您的用例,则可以使用顶点列表创建更自定义的形状。例如,您可能希望创建如下所示的心形
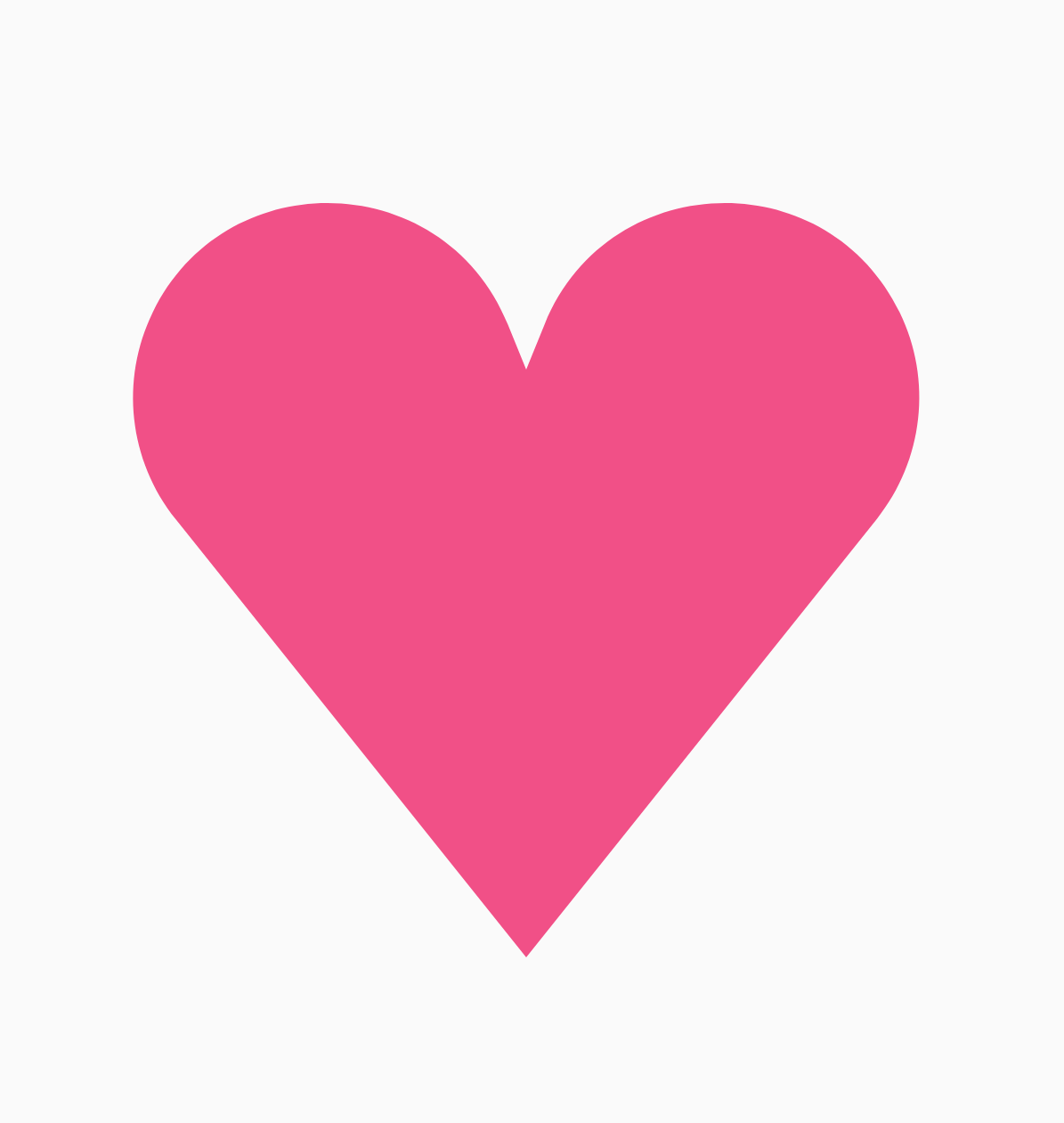
您可以使用接受 x、y 坐标浮点数数组的 RoundedPolygon
重载来指定此形状的各个顶点。
要分解心形多边形,请注意,指定点的极坐标系比使用笛卡尔(x,y)坐标系更容易,其中 0°
从右侧开始,并顺时针进行,270°
在 12 点钟位置
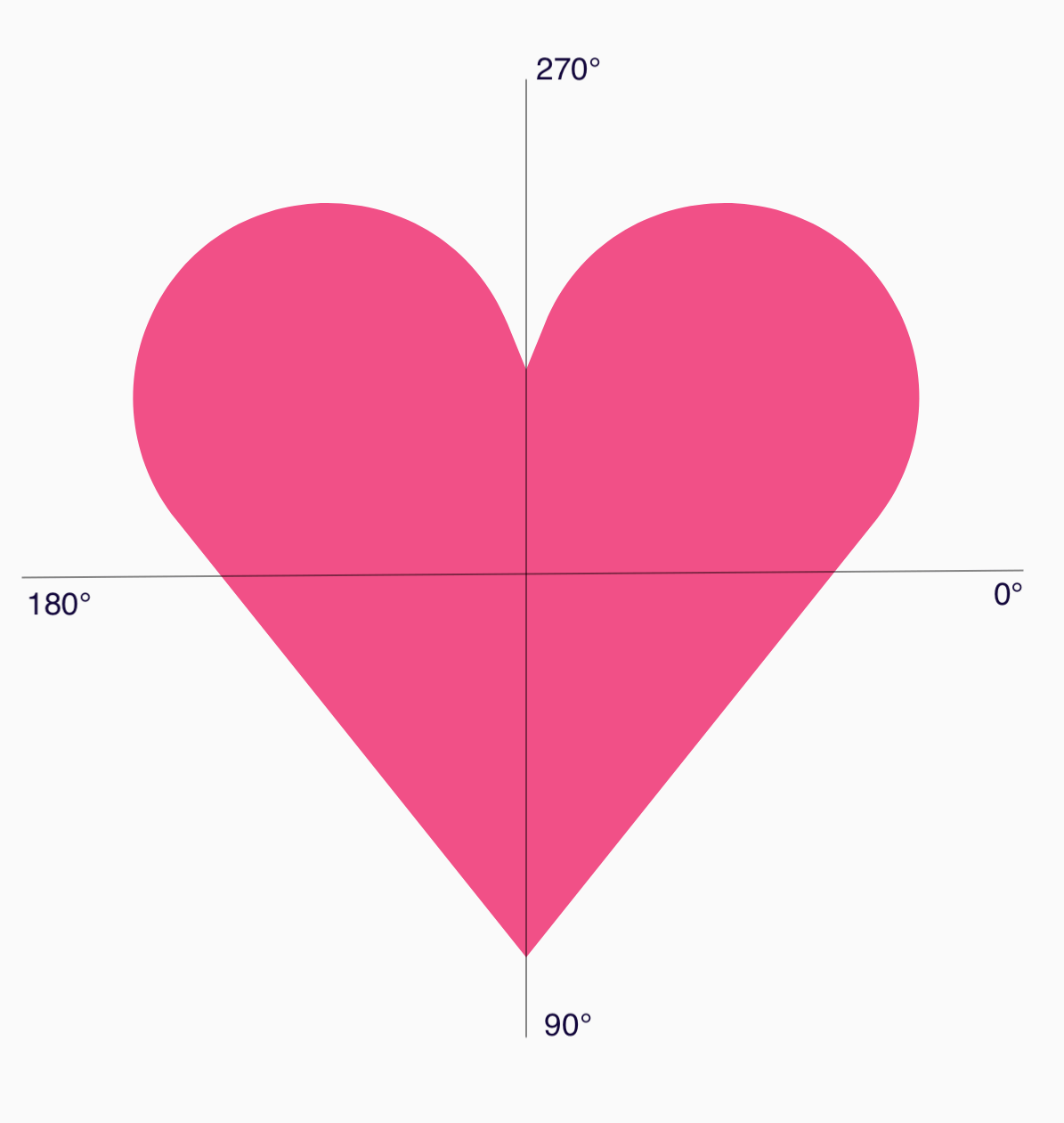
现在可以通过指定每个点处的中心的角度 (𝜭) 和半径以更简单的方式定义形状
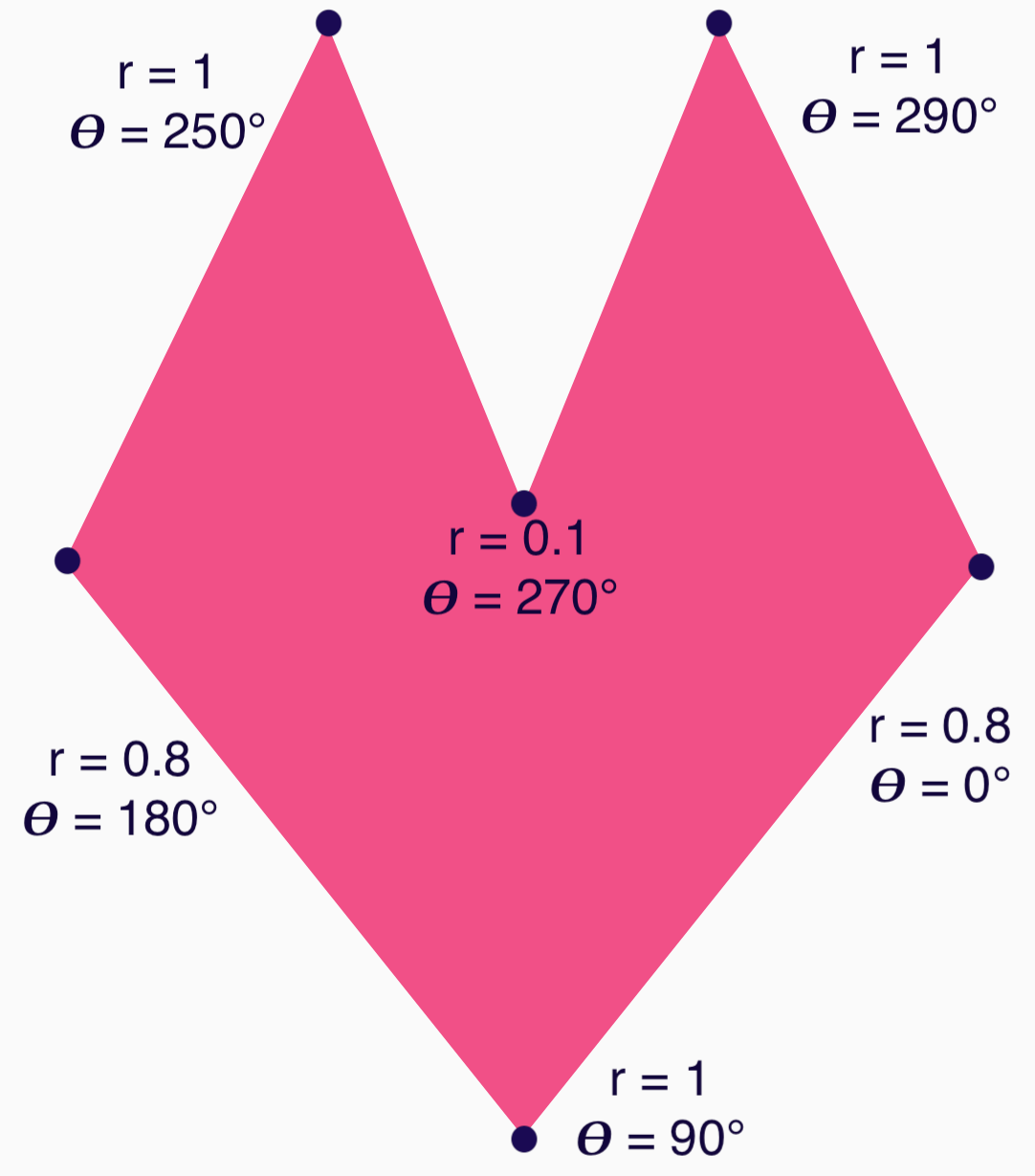
现在可以创建顶点并将其传递给 RoundedPolygon
函数
val vertices = remember { val radius = 1f val radiusSides = 0.8f val innerRadius = .1f floatArrayOf( radialToCartesian(radiusSides, 0f.toRadians()).x, radialToCartesian(radiusSides, 0f.toRadians()).y, radialToCartesian(radius, 90f.toRadians()).x, radialToCartesian(radius, 90f.toRadians()).y, radialToCartesian(radiusSides, 180f.toRadians()).x, radialToCartesian(radiusSides, 180f.toRadians()).y, radialToCartesian(radius, 250f.toRadians()).x, radialToCartesian(radius, 250f.toRadians()).y, radialToCartesian(innerRadius, 270f.toRadians()).x, radialToCartesian(innerRadius, 270f.toRadians()).y, radialToCartesian(radius, 290f.toRadians()).x, radialToCartesian(radius, 290f.toRadians()).y, ) }
需要使用此 radialToCartesian
函数将顶点转换为笛卡尔坐标
internal fun Float.toRadians() = this * PI.toFloat() / 180f internal val PointZero = PointF(0f, 0f) internal fun radialToCartesian( radius: Float, angleRadians: Float, center: PointF = PointZero ) = directionVectorPointF(angleRadians) * radius + center internal fun directionVectorPointF(angleRadians: Float) = PointF(cos(angleRadians), sin(angleRadians))
前面的代码为您提供了心脏的原始顶点,但您需要对特定角进行圆角处理才能获得所需的心形。位于 90°
和 270°
的角没有圆角,但其他角有。要为各个角实现自定义圆角,请使用 perVertexRounding
参数
val rounding = remember { val roundingNormal = 0.6f val roundingNone = 0f listOf( CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), ) } val polygon = remember(vertices, rounding) { RoundedPolygon( vertices = vertices, perVertexRounding = rounding ) } Box( modifier = Modifier .drawWithCache { val roundedPolygonPath = polygon.toPath().asComposePath() onDrawBehind { scale(size.width * 0.5f, size.width * 0.5f) { translate(size.width * 0.5f, size.height * 0.5f) { drawPath(roundedPolygonPath, color = Color(0xFFF15087)) } } } } .size(400.dp) )
这将生成粉红色的心形
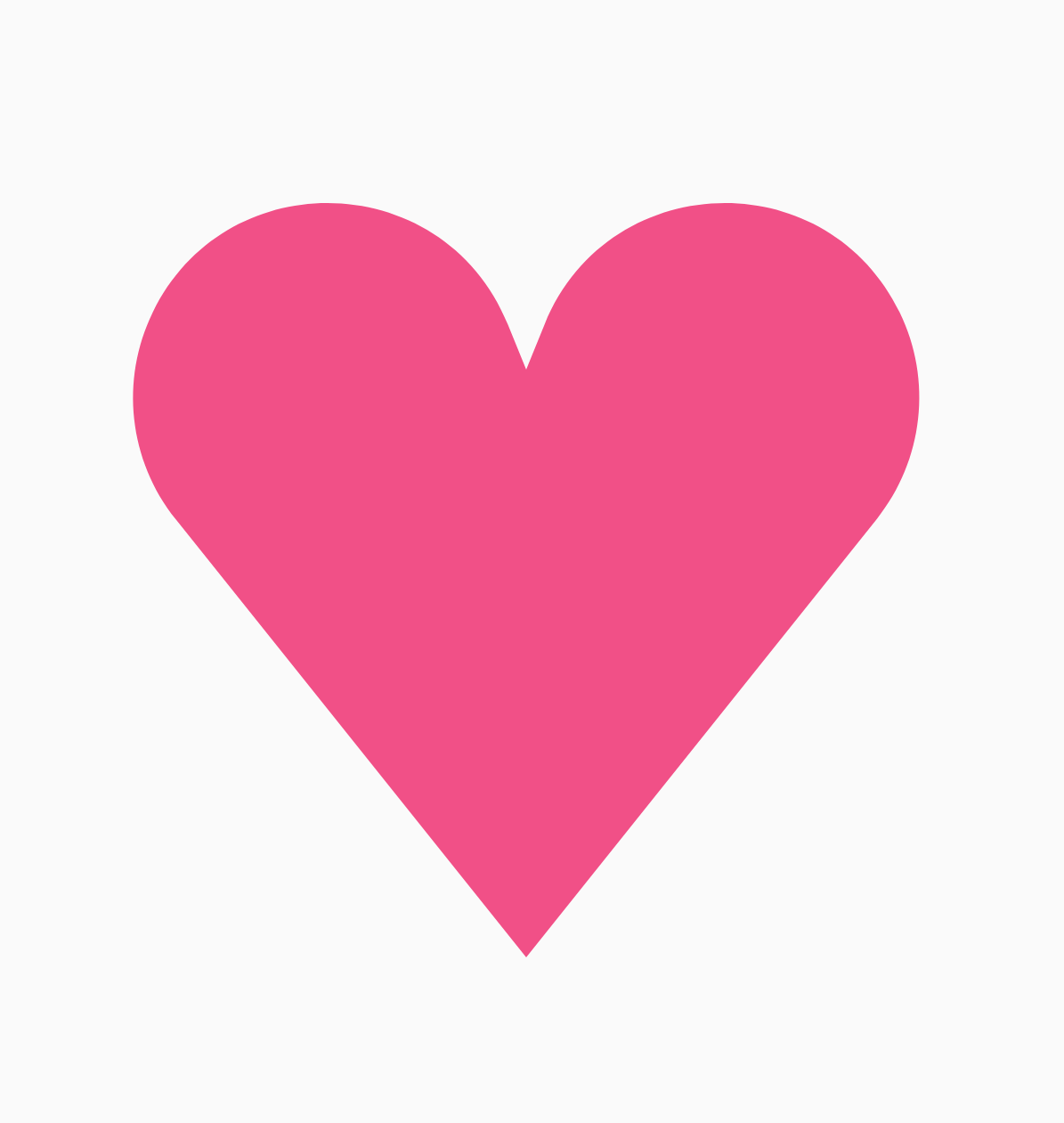
如果前面的形状不符合您的用例,请考虑使用 Path
类来 绘制自定义形状,或从磁盘加载 ImageVector
文件。 graphics-shapes
库并非旨在用于任意形状,而是专门用于简化圆角多边形的创建以及它们之间的变形动画。
其他资源
有关更多信息和示例,请参阅以下资源