此页面介绍如何处理大小以及如何使用现有 Glance 组件使用 Glance 提供灵活且响应迅速的布局。
使用 Box
、Column
和 Row
Glance 有三个主要的可组合布局
Box
:将元素放置在另一个元素之上。它转换为RelativeLayout
。Column
:沿垂直轴将元素一个接一个地放置。它转换为具有垂直方向的LinearLayout
。Row
:沿水平轴将元素一个接一个地放置。它转换为具有水平方向的LinearLayout
。
Glance 支持 Scaffold
对象。将您的 Column
、Row
和 Box
可组合项放置在给定的 Scaffold
对象中。
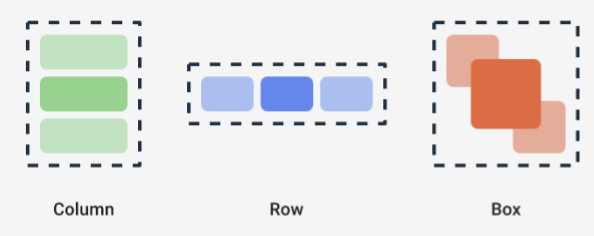
这些可组合项中的每一个都允许您定义其内容的垂直和水平对齐方式以及使用修饰符的宽度、高度、权重或填充约束。此外,每个子项都可以定义其修饰符以更改父项内部的空间和位置。
以下示例演示如何创建一个 Row
,该 Row
在水平方向上均匀分布其子项,如图 1 所示。
Row(modifier = GlanceModifier.fillMaxWidth().padding(16.dp)) { val modifier = GlanceModifier.defaultWeight() Text("first", modifier) Text("second", modifier) Text("third", modifier) }
Row
填充最大可用宽度,并且由于每个子项具有相同的权重,因此它们均匀地共享可用空间。您可以定义不同的权重、大小、填充或对齐方式以使布局适应您的需求。
使用可滚动布局
提供响应式内容的另一种方法是使其可滚动。这可以使用 LazyColumn
可组合项实现。此可组合项允许您定义一组要在应用程序小部件的可滚动容器中显示的项目。
以下代码片段显示了在 LazyColumn
中定义项目 的不同方法。
您可以提供项目数量
// Remember to import Glance Composables // import androidx.glance.appwidget.layout.LazyColumn LazyColumn { items(10) { index: Int -> Text( text = "Item $index", modifier = GlanceModifier.fillMaxWidth() ) } }
提供单个项目
LazyColumn { item { Text("First Item") } item { Text("Second Item") } }
提供项目列表或数组
LazyColumn { items(peopleNameList) { name -> Text(name) } }
您还可以组合使用上述示例
LazyColumn { item { Text("Names:") } items(peopleNameList) { name -> Text(name) } // or in case you need the index: itemsIndexed(peopleNameList) { index, person -> Text("$person at index $index") } }
请注意,前面的代码片段未指定 itemId
。指定 itemId
有助于提高性能并在 Android 12 及更高版本中(例如,在向列表添加或从中删除项目时)维护滚动位置。以下示例演示如何指定 itemId
items(items = peopleList, key = { person -> person.id }) { person -> Text(person.name) }
定义 SizeMode
AppWidget
的大小可能因设备、用户选择或启动器而异,因此如提供灵活的小部件布局页面中所述,提供灵活的布局非常重要。Glance 使用 SizeMode
定义和 LocalSize
值简化了此过程。以下部分介绍三种模式。
SizeMode.Single
SizeMode.Single
是默认模式。它表示仅提供一种类型的内容;也就是说,即使 AppWidget
可用大小发生变化,内容大小也不会改变。
class MyAppWidget : GlanceAppWidget() { override val sizeMode = SizeMode.Single override suspend fun provideGlance(context: Context, id: GlanceId) { // ... provideContent { MyContent() } } @Composable private fun MyContent() { // Size will be the minimum size or resizable // size defined in the App Widget metadata val size = LocalSize.current // ... } }
使用此模式时,请确保
- 根据内容大小正确定义最小和最大大小的元数据值。
- 内容在预期大小范围内足够灵活。
通常,如果以下任一情况成立,则应使用此模式:
a) AppWidget
尺寸固定,或 b) 调整大小后其内容不会改变。
SizeMode.Responsive
此模式相当于提供响应式布局,它允许 GlanceAppWidget
定义一组受特定大小限制的响应式布局。对于每个定义的大小,在创建或更新 AppWidget
时,都会创建内容并将其映射到特定大小。然后,系统会根据可用大小选择最合适的大小。
例如,在我们的目标 AppWidget
中,您可以定义三个大小及其内容
class MyAppWidget : GlanceAppWidget() { companion object { private val SMALL_SQUARE = DpSize(100.dp, 100.dp) private val HORIZONTAL_RECTANGLE = DpSize(250.dp, 100.dp) private val BIG_SQUARE = DpSize(250.dp, 250.dp) } override val sizeMode = SizeMode.Responsive( setOf( SMALL_SQUARE, HORIZONTAL_RECTANGLE, BIG_SQUARE ) ) override suspend fun provideGlance(context: Context, id: GlanceId) { // ... provideContent { MyContent() } } @Composable private fun MyContent() { // Size will be one of the sizes defined above. val size = LocalSize.current Column { if (size.height >= BIG_SQUARE.height) { Text(text = "Where to?", modifier = GlanceModifier.padding(12.dp)) } Row(horizontalAlignment = Alignment.CenterHorizontally) { Button() Button() if (size.width >= HORIZONTAL_RECTANGLE.width) { Button("School") } } if (size.height >= BIG_SQUARE.height) { Text(text = "provided by X") } } } }
在上一个示例中,provideContent
方法被调用三次并映射到定义的大小。
- 在第一次调用中,大小计算为
100x100
。内容不包含额外的按钮,也不包含顶部和底部的文本。 - 在第二次调用中,大小计算为
250x100
。内容包含额外的按钮,但不包含顶部和底部的文本。 - 在第三次调用中,大小计算为
250x250
。内容包含额外的按钮和两个文本。
SizeMode.Responsive
是其他两种模式的组合,它允许您在预定义的边界内定义响应式内容。通常,此模式性能更好,并且在调整 AppWidget
大小时允许更平滑的过渡。
下表显示了根据 SizeMode
和 AppWidget
可用大小而定的尺寸值
可用大小 | 105 x 110 | 203 x 112 | 72 x 72 | 203 x 150 |
---|---|---|---|---|
SizeMode.Single |
110 x 110 | 110 x 110 | 110 x 110 | 110 x 110 |
SizeMode.Exact |
105 x 110 | 203 x 112 | 72 x 72 | 203 x 150 |
SizeMode.Responsive |
80 x 100 | 80 x 100 | 80 x 100 | 150 x 120 |
* 确切的值仅用于演示目的。 |
SizeMode.Exact
SizeMode.Exact
等效于提供精确布局,它每次 AppWidget
可用大小发生变化时(例如,用户在主屏幕上调整 AppWidget
大小时)都会请求 GlanceAppWidget
内容。
例如,在目标小部件中,如果可用宽度大于某个值,则可以添加一个额外的按钮。
class MyAppWidget : GlanceAppWidget() { override val sizeMode = SizeMode.Exact override suspend fun provideGlance(context: Context, id: GlanceId) { // ... provideContent { MyContent() } } @Composable private fun MyContent() { // Size will be the size of the AppWidget val size = LocalSize.current Column { Text(text = "Where to?", modifier = GlanceModifier.padding(12.dp)) Row(horizontalAlignment = Alignment.CenterHorizontally) { Button() Button() if (size.width > 250.dp) { Button("School") } } } } }
此模式比其他模式提供更大的灵活性,但它也有一些注意事项
- 每次大小发生变化时,都必须完全重新创建
AppWidget
。如果内容复杂,这可能会导致性能问题和 UI 跳跃。 - 可用大小可能因启动器的实现而异。例如,如果启动器不提供大小列表,则使用最小可能的大小。
- 在 Android 12 之前的设备上,大小计算逻辑可能并非在所有情况下都能正常工作。
通常,如果无法使用 SizeMode.Responsive
(即,一小组响应式布局不可行),则应使用此模式。
访问资源
使用 LocalContext.current
访问任何 Android 资源,如下例所示
LocalContext.current.getString(R.string.glance_title)
我们建议直接提供资源 ID 以减小最终 RemoteViews
对象的大小并启用动态资源,例如动态颜色。
可组合项和方法使用“提供程序”(例如 ImageProvider
)或使用重载方法(例如 GlanceModifier.background(R.color.blue)
)来接受资源。例如
Column( modifier = GlanceModifier.background(R.color.default_widget_background) ) { /**...*/ } Image( provider = ImageProvider(R.drawable.ic_logo), contentDescription = "My image", )
处理文本
Glance 1.1.0 包含一个用于设置文本样式的 API。使用 fontSize
、fontWeight
或 fontFamily
属性设置 TextStyle 类中的文本样式。
fontFamily
支持所有系统字体,如下例所示,但不支持应用程序中的自定义字体
Text(
style = TextStyle(
fontWeight = FontWeight.Bold,
fontSize = 18.sp,
fontFamily = FontFamily.Monospace
),
text = "Example Text"
)
添加复合按钮
复合按钮是在Android 12 中引入的。Glance 支持以下类型的复合按钮的向后兼容性
这些复合按钮都显示一个可点击的视图,该视图代表“选中”状态。
var isApplesChecked by remember { mutableStateOf(false) } var isEnabledSwitched by remember { mutableStateOf(false) } var isRadioChecked by remember { mutableStateOf(0) } CheckBox( checked = isApplesChecked, onCheckedChange = { isApplesChecked = !isApplesChecked }, text = "Apples" ) Switch( checked = isEnabledSwitched, onCheckedChange = { isEnabledSwitched = !isEnabledSwitched }, text = "Enabled" ) RadioButton( checked = isRadioChecked == 1, onClick = { isRadioChecked = 1 }, text = "Checked" )
状态更改时,将触发提供的 lambda 表达式。您可以存储检查状态,如下例所示
class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { val myRepository = MyRepository.getInstance() provideContent { val scope = rememberCoroutineScope() val saveApple: (Boolean) -> Unit = { scope.launch { myRepository.saveApple(it) } } MyContent(saveApple) } } @Composable private fun MyContent(saveApple: (Boolean) -> Unit) { var isAppleChecked by remember { mutableStateOf(false) } Button( text = "Save", onClick = { saveApple(isAppleChecked) } ) } }
您还可以将 colors
属性提供给 CheckBox
、Switch
和 RadioButton
以自定义其颜色
CheckBox( // ... colors = CheckboxDefaults.colors( checkedColor = ColorProvider(day = colorAccentDay, night = colorAccentNight), uncheckedColor = ColorProvider(day = Color.DarkGray, night = Color.LightGray) ), checked = isChecked, onCheckedChange = { isChecked = !isChecked } ) Switch( // ... colors = SwitchDefaults.colors( checkedThumbColor = ColorProvider(day = Color.Red, night = Color.Cyan), uncheckedThumbColor = ColorProvider(day = Color.Green, night = Color.Magenta), checkedTrackColor = ColorProvider(day = Color.Blue, night = Color.Yellow), uncheckedTrackColor = ColorProvider(day = Color.Magenta, night = Color.Green) ), checked = isChecked, onCheckedChange = { isChecked = !isChecked }, text = "Enabled" ) RadioButton( // ... colors = RadioButtonDefaults.colors( checkedColor = ColorProvider(day = Color.Cyan, night = Color.Yellow), uncheckedColor = ColorProvider(day = Color.Red, night = Color.Blue) ), )
其他组件
Glance 1.1.0 包括其他组件的发布,如下表所述
名称 | 图像 | 参考链接 | 补充说明 |
---|---|---|---|
填充按钮 |
![]() |
组件 | |
轮廓按钮 |
![]() |
组件 | |
图标按钮 |
![]() |
组件 | 主要/次要/仅图标 |
标题栏 |
![]() |
组件 | |
脚手架 | 脚手架和标题栏在同一个演示中。 |
有关设计细节的更多信息,请参阅Figma上的此设计工具包。