AndroidJUnitRunner
类是一个 JUnit 测试运行程序,它允许您在 Android 设备上运行工具 JUnit 4 测试,包括使用 Espresso、UI Automator 和 Compose 测试框架的测试。
测试运行程序负责将您的测试包和被测应用加载到设备,运行您的测试并报告测试结果。
此测试运行程序支持多项常见测试任务,包括以下内容:
编写 JUnit 测试
以下代码片段展示了如何编写工具 JUnit 4 测试以验证 ChangeTextBehavior
类中的 changeText
操作是否正常工作:
Kotlin
@RunWith(AndroidJUnit4::class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation class ChangeTextBehaviorTest { val stringToBeTyped = "Espresso" // ActivityTestRule accesses context through the runner @get:Rule val activityRule = ActivityTestRule(MainActivity::class.java) @Test fun changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()) onView(withId(R.id.changeTextBt)).perform(click()) // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))) } }
Java
@RunWith(AndroidJUnit4.class) // Only needed when mixing JUnit 3 and 4 tests @LargeTest // Optional runner annotation public class ChangeTextBehaviorTest { private static final String stringToBeTyped = "Espresso"; @Rule public ActivityTestRule<MainActivity>; activityRule = new ActivityTestRule<>;(MainActivity.class); @Test public void changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()); onView(withId(R.id.changeTextBt)).perform(click()); // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))); } }
访问应用的上下文
当您使用 AndroidJUnitRunner
运行测试时,您可以通过调用静态 ApplicationProvider.getApplicationContext()
方法来访问被测应用的上下文。如果您在应用中创建了 Application
的自定义子类,则此方法会返回自定义子类的上下文。
如果您是工具实现者,则可以使用InstrumentationRegistry
类访问底层测试API。此类包含Instrumentation
对象、目标应用Context
对象、测试应用Context
对象以及传递到测试中的命令行参数。
筛选测试
在您的JUnit 4.x测试中,您可以使用注解来配置测试运行。此功能最大限度地减少了在测试中添加样板代码和条件代码的需求。除了JUnit 4支持的标准注解外,测试运行程序还支持Android专用注解,包括以下内容:
@RequiresDevice
:指定测试应仅在物理设备上运行,而不是在模拟器上运行。@SdkSuppress
:禁止在低于给定级别的Android API级别上运行测试。例如,要禁止在低于23的所有API级别上运行测试,请使用注解@SDKSuppress(minSdkVersion=23)
。@SmallTest
、@MediumTest
和@LargeTest
:对测试运行所需的时间进行分类,并据此确定可以运行测试的频率。您可以使用此注解来筛选要运行的测试,并设置android.testInstrumentationRunnerArguments.size
属性。
-Pandroid.testInstrumentationRunnerArguments.size=small
分片测试
如果您需要并行执行测试(将测试分散到多个服务器以加快运行速度),则可以将其拆分为组或“分片”。测试运行程序支持将单个测试套件拆分为多个分片,因此您可以轻松地将属于同一分片的测试作为组一起运行。每个分片都由一个索引号标识。运行测试时,使用-e numShards
选项指定要创建的单独分片的数量,并使用-e shardIndex
选项指定要运行的分片。
例如,要将测试套件拆分为10个分片并仅运行第二分片中分组的测试,请使用以下adb命令
adb shell am instrument -w -e numShards 10 -e shardIndex 2
使用Android测试编排器
Android测试编排器允许您在Instrumentation
的每次调用中运行每个应用测试。使用AndroidJUnitRunner 1.0版或更高版本时,您可以访问Android测试编排器。
Android测试编排器为您的测试环境提供了以下好处:
- 共享状态最少:每个测试都在其自己的
Instrumentation
实例中运行。因此,如果您的测试共享应用状态,则大部分共享状态会在每次测试后从设备的CPU或内存中移除。要从设备的CPU和内存中移除每次测试后的所有共享状态,请使用clearPackageData
标志。有关示例,请参阅从Gradle启用部分。 - 崩溃隔离:即使一个测试崩溃,它也只会破坏其自己的
Instrumentation
实例。这意味着套件中的其他测试仍然会运行,从而提供完整的测试结果。
这种隔离可能会导致测试执行时间增加,因为Android测试编排器会在每次测试后重新启动应用程序。
Android Studio和Firebase Test Lab都预安装了Android测试编排器,但您需要在Android Studio中启用此功能。
从Gradle启用
要使用Gradle命令行工具启用Android测试编排器,请完成以下步骤:
- 步骤1:修改gradle文件。将以下语句添加到项目的
build.gradle
文件中:
android {
defaultConfig {
...
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
// The following argument makes the Android Test Orchestrator run its
// "pm clear" command after each test invocation. This command ensures
// that the app's state is completely cleared between tests.
testInstrumentationRunnerArguments clearPackageData: 'true'
}
testOptions {
execution 'ANDROIDX_TEST_ORCHESTRATOR'
}
}
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestUtil 'androidx.test:orchestrator:1.1.0'
}
- 步骤2:通过执行以下命令运行Android测试编排器:
./gradlew connectedCheck
从Android Studio启用
要在Android Studio中启用Android测试编排器,请将从Gradle启用中所示的语句添加到应用的build.gradle
文件中。
从命令行启用
要在命令行上使用Android测试编排器,请在终端窗口中运行以下命令:
DEVICE_API_LEVEL=$(adb shell getprop ro.build.version.sdk)
FORCE_QUERYABLE_OPTION=""
if [[ $DEVICE_API_LEVEL -ge 30 ]]; then
FORCE_QUERYABLE_OPTION="--force-queryable"
fi
# uninstall old versions
adb uninstall androidx.test.services
adb uninstall androidx.test.orchestrator
# Install the test orchestrator.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/orchestrator/1.4.2/orchestrator-1.4.2.apk
# Install test services.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/services/test-services/1.4.2/test-services-1.4.2.apk
# Replace "com.example.test" with the name of the package containing your tests.
# Add "-e clearPackageData true" to clear your app's data in between runs.
adb shell 'CLASSPATH=$(pm path androidx.test.services) app_process / \
androidx.test.services.shellexecutor.ShellMain am instrument -w -e \
targetInstrumentation com.example.test/androidx.test.runner.AndroidJUnitRunner \
androidx.test.orchestrator/.AndroidTestOrchestrator'
如命令语法所示,您安装Android测试编排器,然后直接使用它。
adb shell pm list instrumentation
使用不同的工具链
如果您使用不同的工具链来测试您的应用,您仍然可以通过完成以下步骤来使用Android测试编排器:
架构
编排器服务APK存储在一个与测试APK和被测应用APK分开的进程中。
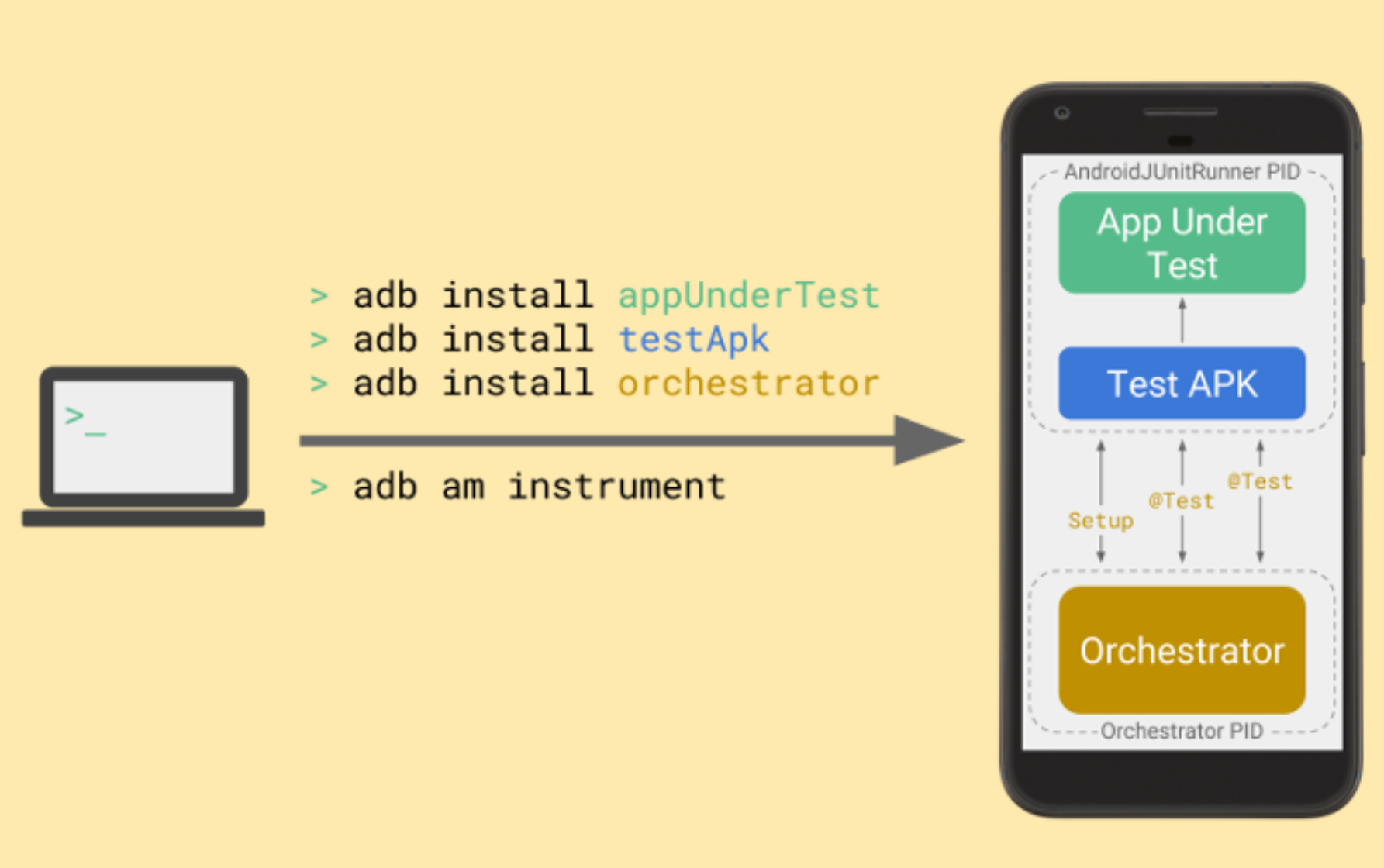
Android测试编排器在测试套件运行开始时收集JUnit测试,但随后会分别在Instrumentation
的自己的实例中执行每个测试。
更多信息
要了解有关使用AndroidJUnitRunner的更多信息,请参阅API参考。
其他资源
有关使用AndroidJUnitRunner
的更多信息,请咨询以下资源。
示例
- AndroidJunitRunnerSample:展示测试注解、参数化测试和测试套件创建。